-3 算术表达式求值(栈)pta本题要实现对输入的表达式进行运算求解,题目为函数补全题,请补全第二部分代码,栈的基本操作,一共包含8个栈操作函数。 补全代码后,将所写代码与题目中已给出的其他部分代码一同提交,运行测试。
时间: 2024-10-19 14:16:34 浏览: 220
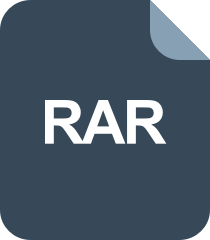
栈实现算术表达式求值和队列实现舞伴配对

在处理-3这样的算术表达式的栈(Stack)求值问题时,通常会使用逆波兰表示法(Reverse Polish Notation,RPN)来简化计算过程。逆波兰表示法不需要括号,而是通过元素顺序来确定运算次序。你需要编写一些基本的栈操作函数,例如入栈、出栈、判断栈是否为空、以及执行两个数的加减乘除等操作。
以下是可能需要补充的栈操作函数示例,假设我们已经有了一个`int`类型的栈`stk`:
```cpp
#include <stack>
#include <string>
class Solution {
public:
// 定义栈数据结构
struct StackElement {
int num;
char op; // '+' '-' '*' '/'
};
std::stack<StackElement> stk;
// 入栈一个操作数
void pushNum(int num) {
StackElement e = {num, 'n'}; // 'n' 表示这是一个数字
stk.push(e);
}
// 出栈并返回顶部的操作数或操作符
int pop() {
if (stk.empty()) return -1; // 栈空处理
StackElement e = stk.top();
stk.pop();
return e.num;
}
// 检查栈是否为空
bool isEmpty() {
return stk.empty();
}
// 出栈并处理操作符,如果遇到数字则入栈,直到遇到另一个操作符或数字结束
void applyOp() {
if (isEmpty()) return;
while (!stk.empty() && (stk.top().op != 'n')) {
int op2 = stk.top().num;
stk.pop(); // 弹出第二个操作数
int op1 = stk.top().num;
stk.pop(); // 弹出第一个操作数
switch(stk.top().op) {
case '+': stk.top().num = op1 + op2; break;
case '-': stk.top().num = op1 - op2; break;
case '*': stk.top().num = op1 * op2; break;
case '/':
if (op2 == 0) throw "Division by zero"; // 防止除零错误
stk.top().num = op1 / op2; break;
default: assert(false); // 错误的操作符
}
}
}
// 主函数用于处理输入的表达式
double calculate(std::string expression) {
for (char c : expression) {
if (isdigit(c)) {
pushNum(c - '0'); // 转换为数字并入栈
} else {
applyOp(); // 当遇到操作符时应用到栈顶的两个操作数
}
}
// 最后一次应用操作符
applyOp();
if (isEmpty()) {
return stk.top().num;
} else {
throw "Invalid expression"; // 栈非空,说明表达式不完整
}
}
};
```
阅读全文
相关推荐
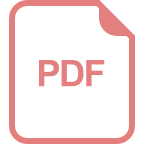
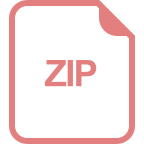
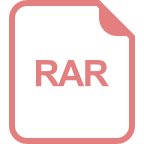
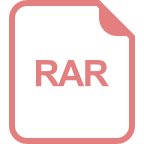
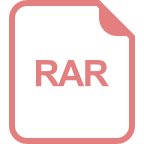
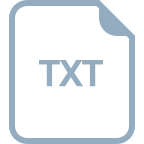
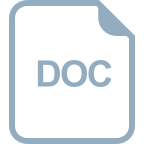
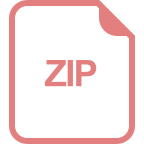
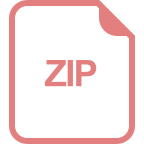
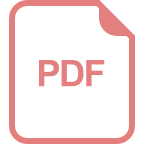
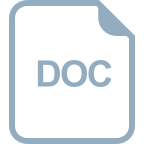
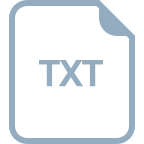
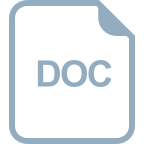