C#判断字符串是否为数字
时间: 2023-11-11 08:51:02 浏览: 105
在C#中,有多种方法可以判断一个字符串是否为数字:
1. 使用Char.IsDigit()方法和Enumerable.All()方法:
```C#
public bool isPureNum(string str)
{
if (str == null || str.Length == 0)
{
return false;
}
bool isNumeric = str.All(char.IsDigit);
return isNumeric;
}
```
2. 使用Regex.IsMatch()方法和正则表达式:
```C#
public bool isPureNum(string str)
{
if (str == null || str.Length == 0)
{
return false;
}
bool isNumeric = System.Text.RegularExpressions.Regex.IsMatch(str, @"^\d$");
return isNumeric;
}
```
3. 使用ASCII码判断:
```C#
public bool isPureNum(string str)
{
if (str == null || str.Length == 0)
{
return false;
}
byte[] strBytes = Encoding.ASCII.GetBytes(str);
foreach (byte strByte in strBytes)
{
if (strByte < 48 || strByte > 57)
{
return false;
}
}
return true;
}
```
4. 使用TryParse()方法:
```C#
public bool isPureNum(string str)
{
if (str == null || str.Length == 0)
{
return false;
}
int num;
bool isNumeric = int.TryParse(str, out num);
return isNumeric;
}
```
相关推荐
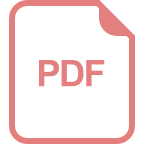














