class HotwordDetector(object): """ Snowboy decoder to detect whether a keyword specified by `decoder_model` exists in a microphone input stream. :param decoder_model: decoder model file path, a string or a list of strings :param resource: resource file path. :param sensitivity: decoder sensitivity, a float of a list of floats. The bigger the value, the more senstive the decoder. If an empty list is provided, then the default sensitivity in the model will be used. :param audio_gain: multiply input volume by this factor. :param apply_frontend: applies the frontend processing algorithm if True. """ def __init__(self, decoder_model, resource=RESOURCE_FILE, sensitivity=[], audio_gain=1, apply_frontend=False): tm = type(decoder_model) ts = type(sensitivity) if tm is not list: decoder_model = [decoder_model] if ts is not list: sensitivity = [sensitivity] model_str = ",".join(decoder_model) self.detector = snowboydetect.SnowboyDetect( resource_filename=resource.encode(), model_str=model_str.encode()) self.detector.SetAudioGain(audio_gain) self.detector.ApplyFrontend(apply_frontend) self.num_hotwords = self.detector.NumHotwords() if len(decoder_model) > 1 and len(sensitivity) == 1: sensitivity = sensitivity * self.num_hotwords if len(sensitivity) != 0: assert self.num_hotwords == len(sensitivity), \ "number of hotwords in decoder_model (%d) and sensitivity " \ "(%d) does not match" % (self.num_hotwords, len(sensitivity)) sensitivity_str = ",".join([str(t) for t in sensitivity]) if len(sensitivity) != 0: self.detector.SetSensitivity(sensitivity_str.encode()) self.ring_buffer = RingBuffer( self.detector.NumChannels() * self.detector.SampleRate() * 5) def start(self, detected_callback=play_audio_file, interrupt_check=lambda: False, sleep_time=0.03, audio_recorder_callback=None, silent_count_threshold=15, recording_timeout=100):
时间: 2024-02-10 10:17:54 浏览: 26
这段代码看起来像是一个热词检测器类的初始化方法。参数包括 `decoder_model`,一个解码器模型文件的路径;`resource`,资源文件的路径;`sensitivity`,一个或多个检测器灵敏度的浮点数;`audio_gain`,音频增益的倍数;`apply_frontend`,是否应用前端处理算法。这个类的实例化会生成一个 SnowboyDetect 对象,用于检测语音流中是否存在指定的关键词。类还包括了一些方法,用于开始检测、回调处理、中断检查等。
相关问题
ModuleNotFoundError: No module named '_snowboydetect'
这个错误通常表示你的环境缺少 `snowboy` 模块。`snowboy` 是一个用于语音唤醒的 Python 模块,它可以用来在自定义语音助手中实现唤醒功能。
要解决这个问题,你可以尝试以下步骤:
1. 确保你已经安装了 `snowboy` 模块。你可以使用 pip 命令来安装它:
```
pip install snowboy
```
2. 如果你已经安装了 `snowboy`,但仍然出现错误,请尝试重新安装它:
```
pip uninstall snowboy
pip install snowboy
```
3. 如果你使用的是虚拟环境,请确保你已经激活了正确的环境。
4. 如果上述方法都没有解决问题,那可能是因为你的系统缺少一些依赖库。根据你的操作系统和环境,你可以查看 `snowboy` 的官方文档或者 GitHub 页面寻找解决方案。
希望这些步骤能够帮助你解决问题!如果还有其他疑问,请随时提问。
python3 demo.py resources/models/smart_mirror.umdl Traceback (most recent call last): File "/home/pi/snowboy/examples/Python3/demo.py", line 1, in <module> import snowboydecoder File "/home/pi/snowboy/examples/Python3/snowboydecoder.py", line 5, in <module> import snowboydetect File "/home/pi/snowboy/examples/Python3/snowboydetect.py", line 15, in <module> import _snowboydetect ModuleNotFoundError: No module named '_snowboydetect'
看起来你在尝试运行一个使用Snowboy唤醒词检测库的Python脚本,但是出现了一个名为"_snowboydetect"的未找到模块的错误。这通常是因为您需要安装Snowboy的C++库并编译Python绑定才能使用Snowboy的Python库。请确保您已按照Snowboy文档中的说明正确地安装Snowboy,包括编译Python绑定,并且您的Python环境已正确配置。
相关推荐
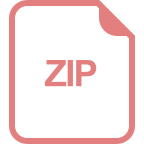
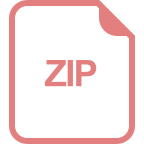
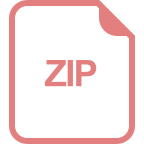












