Java多线程编程学习笔记之十二:生产者—消费者模型(含代码)
时间: 2023-11-16 07:07:59 浏览: 149
好的,下面是Java多线程编程学习笔记之十二:生产者—消费者模型的相关内容和代码。
## 生产者—消费者模型简介
生产者—消费者模型是一种常见的多线程并发模型,它涉及到两个角色:生产者和消费者。生产者负责生产数据,消费者负责消费数据。生产者和消费者通过一个共享的缓冲区进行通信,生产者将数据放入缓冲区,消费者从缓冲区获取数据。
在多线程编程中,生产者—消费者模型的实现有多种方式,本文将介绍一种基于Java的实现方式。
## 生产者—消费者模型的实现
### 1. 定义共享缓冲区
共享缓冲区是生产者和消费者进行通信的桥梁,它需要实现以下功能:
- 提供一个put方法,允许生产者将数据放入缓冲区;
- 提供一个take方法,允许消费者从缓冲区获取数据;
- 当缓冲区已满时,put方法应该等待;
- 当缓冲区为空时,take方法应该等待。
以下是一个简单的共享缓冲区的实现:
```java
public class Buffer {
private int[] data;
private int size;
private int count;
private int putIndex;
private int takeIndex;
public Buffer(int size) {
this.data = new int[size];
this.size = size;
this.count = 0;
this.putIndex = 0;
this.takeIndex = 0;
}
public synchronized void put(int value) throws InterruptedException {
while (count == size) {
wait();
}
data[putIndex] = value;
putIndex = (putIndex + 1) % size;
count++;
notifyAll();
}
public synchronized int take() throws InterruptedException {
while (count == 0) {
wait();
}
int value = data[takeIndex];
takeIndex = (takeIndex + 1) % size;
count--;
notifyAll();
return value;
}
}
```
上面的Buffer类使用一个数组来表示缓冲区,size表示缓冲区的大小,count表示当前缓冲区中的元素数量,putIndex和takeIndex分别表示下一个可写和可读的位置。put和take方法都是同步方法,使用wait和notifyAll来进行线程间的等待和通知。
### 2. 定义生产者和消费者
生产者和消费者都需要访问共享缓冲区,因此它们都需要接收一个Buffer对象作为参数。以下是生产者和消费者的简单实现:
```java
public class Producer implements Runnable {
private Buffer buffer;
public Producer(Buffer buffer) {
this.buffer = buffer;
}
public void run() {
try {
for (int i = 0; i < 10; i++) {
buffer.put(i);
System.out.println("Produced: " + i);
Thread.sleep((int)(Math.random() * 1000));
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class Consumer implements Runnable {
private Buffer buffer;
public Consumer(Buffer buffer) {
this.buffer = buffer;
}
public void run() {
try {
for (int i = 0; i < 10; i++) {
int value = buffer.take();
System.out.println("Consumed: " + value);
Thread.sleep((int)(Math.random() * 1000));
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
生产者在一个循环中不断地向缓冲区中放入数据,消费者也在一个循环中不断地从缓冲区中获取数据。注意,当缓冲区已满时,生产者会进入等待状态;当缓冲区为空时,消费者会进入等待状态。
### 3. 测试
最后,我们可以使用下面的代码来进行测试:
```java
public class Main {
public static void main(String[] args) {
Buffer buffer = new Buffer(5);
Producer producer = new Producer(buffer);
Consumer consumer = new Consumer(buffer);
Thread producerThread = new Thread(producer);
Thread consumerThread = new Thread(consumer);
producerThread.start();
consumerThread.start();
}
}
```
在上面的代码中,我们创建了一个缓冲区对象和一个生产者对象和一个消费者对象,然后将它们分别传递给两个线程,并启动这两个线程。
运行上面的代码,我们可以看到生产者和消费者交替地进行操作,生产者不断地向缓冲区中放入数据,消费者不断地从缓冲区中获取数据。如果缓冲区已满或者为空,生产者和消费者会进入等待状态,直到缓冲区中有足够的空间或者有新的数据可用。
相关推荐
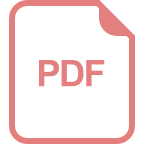














