在flutter中如何通过本地存储保存状态,有两个页面,第二个页面定义了初始isShow为false,也有一个改变isShow状态的按钮,第一个页面进去第二个页面时初始isShow为false,通过点击按钮改变isShow为true,回到第一个页面后重新进入第二个页面时,为什么isShow还是为false,如何保存isShow为按钮点击后的isShow状态,如何进入时为true
时间: 2024-02-13 13:04:49 浏览: 25
在Flutter中,您可以使用shared_preferences插件来实现本地存储来保存状态。以下是实现您所描述的场景的示例代码:
在第二个页面中,您可以使用SharedPreferences来存储isShow状态,如下所示:
```
import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
class SecondPage extends StatefulWidget {
@override
_SecondPageState createState() => _SecondPageState();
}
class _SecondPageState extends State<SecondPage> {
bool isShow;
@override
void initState() {
super.initState();
// 从SharedPreferences中获取isShow状态,如果为空则默认为false
SharedPreferences.getInstance().then((prefs) {
setState(() {
isShow = prefs.getBool('isShow') ?? false;
});
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Second Page'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(isShow ? 'Is Show' : 'Not Show'),
SizedBox(height: 16),
ElevatedButton(
child: Text('Toggle'),
onPressed: () {
setState(() {
isShow = !isShow;
});
// 将isShow状态保存到SharedPreferences中
SharedPreferences.getInstance().then((prefs) {
prefs.setBool('isShow', isShow);
});
},
),
],
),
),
);
}
}
```
在第一个页面中,您可以使用Navigator.pushNamedAndRemoveUntil来跳转到第二个页面,并在返回时清除路由历史记录,以便再次进入第二个页面时重新加载isShow状态。以下是示例代码:
```
import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
import 'second_page.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
initialRoute: '/',
routes: {
'/': (context) => HomePage(),
'/second': (context) => SecondPage(),
},
);
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Home Page'),
),
body: Center(
child: ElevatedButton(
child: Text('Go to Second Page'),
onPressed: () async {
// 清除路由历史记录
await Navigator.pushNamedAndRemoveUntil(
context,
'/second',
(route) => false,
);
// 重新获取isShow状态并更新页面
SharedPreferences prefs = await SharedPreferences.getInstance();
bool isShow = prefs.getBool('isShow') ?? false;
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text('isShow: $isShow')),
);
},
),
),
);
}
}
```
在上面的示例代码中,当用户从第二个页面返回时,它将获取SharedPreferences中存储的isShow状态,并在Snackbar中显示它。
希望这能回答您的问题!
相关推荐
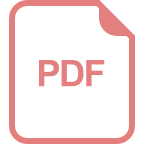
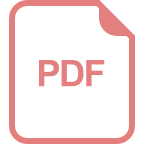
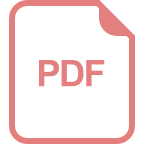














