使用C++编写一个从CSV文件中读取数据的代码
时间: 2023-05-24 11:00:58 浏览: 87
Sure, I can help with that! Here is some sample C code to read data from a CSV file:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LENGTH 1024
#define MAX_NUM_FIELDS 10
int main()
{
char filename[] = "data.csv";
char line[MAX_LINE_LENGTH];
char* field[MAX_NUM_FIELDS];
int num_fields;
FILE* file = fopen(filename, "r");
if (file == NULL) {
printf("Failed to open file: %s\n", filename);
return 1;
}
while (fgets(line, sizeof(line), file)) {
num_fields = 0;
field[num_fields] = strtok(line, ",");
while (field[num_fields] != NULL && num_fields < MAX_NUM_FIELDS) {
num_fields++;
field[num_fields] = strtok(NULL, ",");
}
// Do something with the fields
for (int i = 0; i < num_fields; i++) {
printf("Field %d: %s\n", i, field[i]);
}
}
fclose(file);
return 0;
}
```
This code opens a CSV file named `data.csv`, reads each line of the file, and splits it into separate fields using the comma as a delimiter. The fields are then printed to the console. You can modify the code to do whatever you need with the data.
I hope this helps! Let me know if you have any other questions.
阅读全文
相关推荐


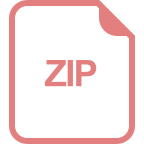
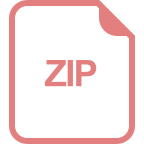
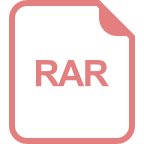
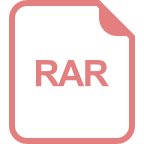
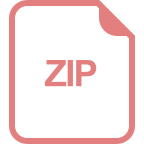
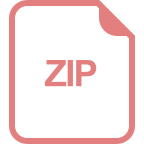







