C#仿真一个点在一个线段上的轨迹,点以不同的速度在线段上运行,使用Form来体现出来
时间: 2024-04-14 19:25:59 浏览: 95
要在C#中使用Windows Forms来模拟一个点在线段上的轨迹,可以创建一个Windows Forms应用程序,并使用Timer和Graphics对象来实现点的运动和绘制。以下是一个示例代码:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
class LineSegment
{
public Point startPoint;
public Point endPoint;
public LineSegment(Point startPoint, Point endPoint)
{
this.startPoint = startPoint;
this.endPoint = endPoint;
}
public void Draw(Graphics g)
{
g.DrawLine(Pens.Black, startPoint, endPoint);
}
}
class SimulationForm : Form
{
private LineSegment line;
private Point currentPoint;
private double speed;
private double t;
private Timer timer;
public SimulationForm()
{
line = new LineSegment(new Point(50, 50), new Point(200, 200));
currentPoint = line.startPoint;
speed = 0.01; // 点的速度,可以根据需要调整
timer = new Timer();
timer.Interval = 10; // 定时器的间隔时间,可以根据需要调整
timer.Tick += Timer_Tick;
timer.Start();
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
Graphics g = e.Graphics;
line.Draw(g);
g.FillEllipse(Brushes.Red, currentPoint.X - 5, currentPoint.Y - 5, 10, 10);
}
private void Timer_Tick(object sender, EventArgs e)
{
t += speed;
if (t > 1)
{
t = 1;
timer.Stop();
timer.Dispose();
}
int x = (int)(line.startPoint.X + t * (line.endPoint.X - line.startPoint.X)); // 计算x坐标
int y = (int)(line.startPoint.Y + t * (line.endPoint.Y - line.startPoint.Y)); // 计算y坐标
currentPoint = new Point(x, y);
Invalidate(); // 刷新窗体
}
}
class Program
{
static void Main(string[] args)
{
Application.Run(new SimulationForm());
}
}
```
在上面的示例中,我们创建了一个继承自Form的SimulationForm类来表示仿真窗体。在构造函数中,我们定义了一个LineSegment对象来表示线段,并初始化当前点为线段的起点。我们还创建了一个Timer对象来定时更新点的位置。在OnPaint方法中,我们使用Graphics对象绘制线段和当前点。在Timer_Tick事件处理程序中,我们根据速度参数t计算点的位置,并更新当前点的坐标。当t大于1时,表示点已经到达线段的终点,我们停止并释放定时器。每次更新后,我们调用Invalidate方法刷新窗体,以便重新绘制。
你可以根据需要调整线段的起点、终点、速度和定时器的间隔时间。运行程序后,你将看到一个窗体中绘制了线段,并模拟了点在线段上以不同速度运动的轨迹。
阅读全文
相关推荐
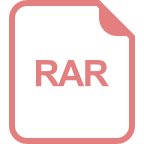
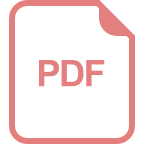


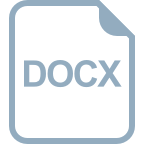
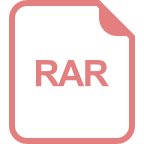
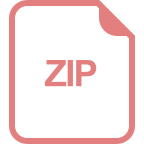
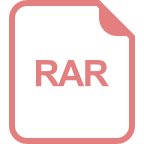
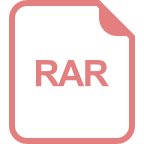
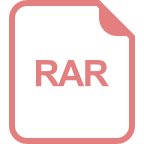
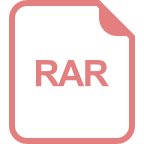
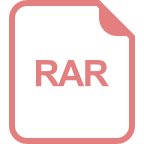
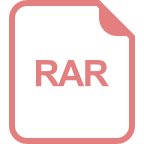
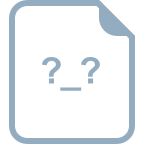