@Controller @RequestMapping("/zmu") public class LoginController { @Autowired AccountDao adao; @GetMapping("/login") public String login(){ return "login"; } @GetMapping("/registry") public String registry(){ return "registry"; } @PostMapping("/addaccount") //方法中的参数名一定要与网页端属性为name的值一致 public String addAccount(String username,String password, Model model){ Account account=new Account(username,password); account= adao.saveAndFlush(account); if(account.getId()>0) model.addAttribute("success","帐户和密码添加成功"); else model.addAttribute("success","添加帐户和密码失败"); //redirect跳转到对路径,所带的信息会被丢弃 //redirect:/ return "registry"; } @PostMapping("/verify") //方法中的参数名一定要与网页端属性为name的值一致 public String verify(String username,String password, Model model){ boolean s=adao.existsByUsernameAndPassword(username,password); if(s) model.addAttribute("success","合法用户验证成功"); else model.addAttribute("success","无此帐户和密码,登录失败"); //redirect跳转到对路径,所带的信息会被丢弃 //redirect:/ return "login"; } //浏览帐户信息 @GetMapping("/accountshow") public String accountshow(Model model){ List<Account> lists=adao.findAll(); if(lists.size()!=0){ model.addAttribute("success",true); model.addAttribute("account",lists); } else model.addAttribute("success",false); return "account_show"; }对应的删除用户的方法代码
时间: 2023-06-26 10:09:08 浏览: 88
@PostMapping("/deleteaccount")
public String deleteAccount(int id, Model model){
try{
adao.deleteById(id);
model.addAttribute("success","删除帐户成功");
}catch(Exception e){
model.addAttribute("success","删除帐户失败");
}
return "account_show";
}
相关问题
详细注释下列代码:@Controller public class LoginController { @Autowired private UserService userService; @RequestMapping("/login/login") public String login(){ return "login"; } @RequestMapping("/login") public String login1(){ return "login"; } @ResponseBody @RequestMapping("/login/index") public Msg loginIndex(User user,HttpSession httpSession){ User user1=userService.findUserByUsername(user.getUsername()); if(user1.getPassword().equals(user.getPassword())) { httpSession.setAttribute("user", user1); return Msg.success(); }else{ return Msg.fail().add("va_msg", "密码错误"); } } //ajax校验username是否存在 @ResponseBody @RequestMapping("/login/checkUsernameExit") public Msg checkUsernameExit(@RequestParam("username")String username){ System.out.println("username:"+username); User user=userService.findUserByUsername(username); if(user==null) { return Msg.fail().add("va_msg", "用户名不存在"); } return Msg.success(); } }
这段代码是一个Java类,使用了@Controller注解来表示这是一个控制层的类。其中@Autowired注解用于进行依赖注入,将UserService对象注入到该类中。该类包含了多个请求映射的方法,每个方法的@RequestMapping注解指定了该方法对应的请求路径。其中,login方法的@RequestMapping注解为"/login/login",指定了登录页面的路径。login1方法的@RequestMapping注解为"/login",指定了登录操作的路径。loginIndex方法的@ResponseBody和@RequestMapping注解指定了登录验证的路径,并将返回值封装为一个Msg对象。在方法中,首先通过调用UserService中的findUserByUsername方法来查找指定用户名的用户,若用户存在且密码正确,则将用户对象保存在Session中,并返回成功信息。否则返回失败信息并提示密码错误。最后一个方法是用于ajax校验用户名是否存在的方法,其中@RequestParam注解指定了传入的参数名,该方法返回一个Msg对象,如果用户不存在则返回失败信息并提示用户名不存在,否则返回成功信息。
下面代码会出现什么问题,如何解决? Java @RestController @RequestMapping("/api") public class MyController { @Autowired private MyService service; @GetMapping("/foo") public String foo() { return service.doSomething(); } } @Service public class MyService { @Autowired private SomeOtherService otherService; public String doSomething() { return otherService.getSomething(); } } public class SomeOtherService { public String getSomething() { return "something"; } }
这段代码的问题是MyService类没有使用@Service注解进行标注,Spring容器无法识别该类为一个Bean,无法进行自动注入。要解决这个问题,只需要在MyService类上添加@Service注解即可。
阅读全文
相关推荐
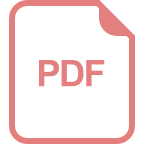
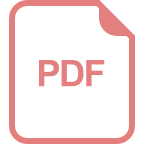
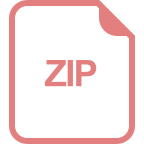
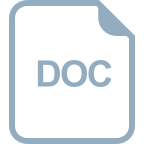








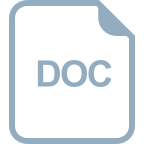



