python写简单农场小游戏
时间: 2023-06-29 08:20:06 浏览: 64
好的,下面是一个简单的农场小游戏示例:
```python
import random
class Crop:
def __init__(self, growth_rate, water_need, light_need):
self._growth = 0
self._days_growing = 0
self._growth_rate = growth_rate
self._water_need = water_need
self._light_need = light_need
self._status = "Seed"
self._type = "Generic"
def needs(self):
return {"Water": self._water_need, "Light": self._light_need}
def report(self):
return {"Type": self._type, "Status": self._status, "Growth": self._growth, "Days growing": self._days_growing}
def _update_status(self):
if self._growth >= 15:
self._status = "Old"
elif self._growth >= 10:
self._status = "Mature"
elif self._growth >= 5:
self._status = "Growing"
elif self._growth >= 0:
self._status = "Seed"
def grow(self, water, light):
if water >= self._water_need and light >= self._light_need:
self._growth += self._growth_rate
self._days_growing += 1
self._update_status()
class Wheat(Crop):
def __init__(self):
super().__init__(1, 3, 6)
self._type = "Wheat"
class Potato(Crop):
def __init__(self):
super().__init__(2, 4, 8)
self._type = "Potato"
class Farm:
def __init__(self, crops):
self._crops = crops
def grow_crops(self, water, light):
for crop in self._crops:
crop.grow(water, light)
def report_crops(self):
reports = []
for crop in self._crops:
reports.append(crop.report())
return reports
def harvest_crop(self, crop_type):
for crop in self._crops:
if isinstance(crop, crop_type) and crop._status == "Mature":
self._crops.remove(crop)
return crop
return None
class Game:
def __init__(self):
self._farm = Farm([Wheat(), Wheat(), Potato(), Potato()])
self._water = 10
self._light = 10
def play(self):
while True:
print("Water level: {}".format(self._water))
print("Light level: {}".format(self._light))
print("1. Grow crops")
print("2. Harvest wheat")
print("3. Harvest potatoes")
print("4. End day")
choice = input("Enter choice: ")
if choice == "1":
self._farm.grow_crops(self._water, self._light)
print("Crops have grown!")
elif choice == "2":
wheat = self._farm.harvest_crop(Wheat)
if wheat:
print("You harvested some wheat!")
else:
print("No wheat is ready for harvest.")
elif choice == "3":
potato = self._farm.harvest_crop(Potato)
if potato:
print("You harvested some potatoes!")
else:
print("No potatoes are ready for harvest.")
elif choice == "4":
self._water -= 1
self._light -= 1
self._farm.grow_crops(self._water, self._light)
reports = self._farm.report_crops()
for report in reports:
print(report)
if self._water <= 0 or self._light <= 0:
print("Game over!")
return
if random.randint(0, 100) < 10:
print("A rain storm has hit!")
self._water += 5
if random.randint(0, 100) < 10:
print("A heat wave has hit!")
self._light += 5
else:
print("Invalid choice.")
if __name__ == "__main__":
game = Game()
game.play()
```
在上面的代码中,我们定义了三个类:Crop、Wheat和Potato。Crop是一个基类,而Wheat和Potato是Crop的子类。我们还定义了一个Farm类和一个Game类。
Game类是我们的游戏主类。它包含一个Farm对象和游戏的主要循环。在每个循环中,玩家可以选择四种不同的操作:生长作物、收获小麦、收获土豆和结束一天。每个操作都会影响玩家的水和光等级,并且可能会触发随机事件,例如暴风雨或热浪。
Farm类包含一组作物。它有一个grow_crops方法,用于生长所有作物,并且有一个report_crops方法,用于打印每个作物的状态。它还有一个harvest_crop方法,用于收获特定类型的作物。
Crop类是作物的基类。它有一些属性,例如_growth和_days_growing,用于追踪作物的成长过程。它还有一些方法,例如needs、report和grow,这些方法用于管理作物的需求和状态。Wheat和Potato类是Crop的子类,它们分别代表小麦和土豆作物。它们覆盖了一些Crop的方法,并定义了自己的特定需求和状态。
相关推荐
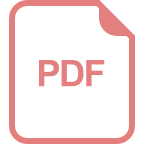








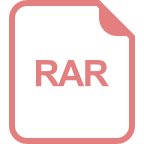
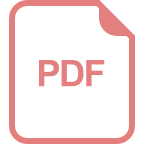
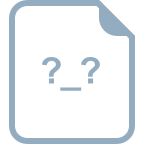
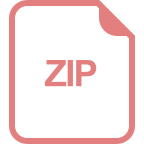