用STM32F103C8T6单片机编写一套基于gsm收发短信控制继电器的程序
时间: 2024-04-09 07:29:20 浏览: 28
以下是使用STM32F103C8T6单片机编写基于GSM收发短信控制继电器的简单示例程序:
```c
#include "stm32f10x.h"
#include "stdio.h"
#include "string.h"
#define USART1_BAUDRATE 9600
void USART1_Init()
{
GPIO_InitTypeDef GPIO_InitStruct;
USART_InitTypeDef USART_InitStruct;
// Enable GPIOA and USART1 clocks
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
// Configure USART1 TX pin (PA9) as alternate function push-pull
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStruct);
// Configure USART1 RX pin (PA10) as input floating
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStruct);
// Configure USART1
USART_InitStruct.USART_BaudRate = USART1_BAUDRATE;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStruct);
// Enable USART1
USART_Cmd(USART1, ENABLE);
}
void GSM_SendCommand(char* command)
{
while (*command)
{
USART_SendData(USART1, *command++);
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
}
}
void GSM_Init()
{
// Configure GSM module baudrate
GSM_SendCommand("AT+IPR=9600\r\n");
delay_ms(100);
// Set GSM module to text mode
GSM_SendCommand("AT+CMGF=1\r\n");
delay_ms(100);
}
void GSM_SendSMS(char* phoneNumber, char* message)
{
char command[50];
// Set phone number
sprintf(command, "AT+CMGS=\"%s\"\r\n", phoneNumber);
GSM_SendCommand(command);
delay_ms(100);
// Set message content
GSM_SendCommand(message);
delay_ms(100);
// Send message
USART_SendData(USART1, 0x1A);
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
delay_ms(1000);
}
int main(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitTypeDef GPIO_InitStruct;
// Configure GPIOC Pin 13 as output push-pull
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_13;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOC, &GPIO_InitStruct);
USART1_Init();
GSM_Init();
while (1)
{
// Check for new SMS
GSM_SendCommand("AT+CMGL=\"REC UNREAD\"\r\n");
delay_ms(2000);
// Process received SMS
// ...
// Control the relay based on the received SMS
// Example: If the received SMS is "ON", turn on the relay
// If the received SMS is "OFF", turn off the relay
// ...
}
}
```
请注意,上述代码仅为示例,可能需要根据您的具体硬件和电路连接进行适当修改。同时,GSM模块的具体型号和AT指令也需要根据实际情况进行相应的调整。此外,您可能还需要实现一个延时函数 `delay_ms()` 来提供延时功能。
相关推荐
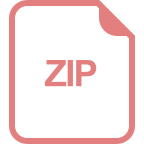
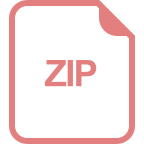
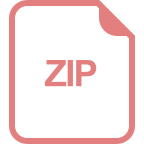














