#include <iostream> #include <cstring> #include <algorithm> #include <vector> using namespace std; const int N = 10; int n, m; int a[N]; int main() { cout << "请输入结点数:" << endl; while (cin >> n >> m) //输入图1、2的结点数 { if (m != n) { cout << "两图不同构!!!" << endl; continue; } //根据结点数n、m,动态生成两个二维数组来存图(使用邻接矩阵的方式) vector<int> w1(n + 1, 0); vector<vector<int> > g1(n + 1, w1); vector<int> w2(m + 1, 0); vector<vector<int> > g2(m + 1, w2); for (int i = 1; i <= n; i++) a[i] = i; //初始化a数组 for (int i = 1; i <= n; i++) //输入图1的邻接矩阵 for (int j = 1; j <= n; j++) cin >> g1[i][j]; for (int i = 1; i <= m; i++) //输入图2的邻接矩阵 for (int j = 1; j <= m; j++) cin >> g2[i][j]; //全排列 do { bool f = 0; int temp[N][N]; memset(temp, 0, sizeof temp); for (int i = 1; i <= n; i++) { for (int j = 1; j <= n; j++) { temp[i][j] = g1[a[i]][a[j]]; } } for (int i = 1; i <= n; i++) { for (int j = 1; j <= n; j++) { if (temp[i][j] != g2[i][j]) { f = 1; break; } } if (f == 1) break; } if (f == 0) cout << "两图同构^-^" << endl; } while (next_permutation(a + 1, a + 1 + n)); cout << "两图不同构!!!" << endl; } return 0; }简化的完整代码
时间: 2024-01-13 12:03:39 浏览: 70
这是一个判断两个图是否同构的程序,输入两个图的邻接矩阵,通过枚举两个图的节点的排列顺序,判断它们是否同构。如果是同构的,则输出"两图同构^-^",否则输出"两图不同构!!!"。
```cpp
#include <iostream>
#include <cstring>
#include <algorithm>
#include <vector>
using namespace std;
const int N = 10;
int n, m;
int a[N];
int main() {
cout << "请输入结点数:" << endl;
while (cin >> n >> m) { //输入图1、2的结点数
if (m != n) {
cout << "两图不同构!!!" << endl;
continue;
}
//根据结点数n、m,动态生成两个二维数组来存图(使用邻接矩阵的方式)
vector<int> w1(n + 1, 0);
vector<vector<int> > g1(n + 1, w1);
vector<int> w2(m + 1, 0);
vector<vector<int> > g2(m + 1, w2);
for (int i = 1; i <= n; i++) a[i] = i; //初始化a数组
for (int i = 1; i <= n; i++) //输入图1的邻接矩阵
for (int j = 1; j <= n; j++)
cin >> g1[i][j];
for (int i = 1; i <= m; i++) //输入图2的邻接矩阵
for (int j = 1; j <= m; j++)
cin >> g2[i][j];
//全排列
do {
bool f = 0;
int temp[N][N];
memset(temp, 0, sizeof temp);
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n; j++) {
temp[i][j] = g1[a[i]][a[j]];
}
}
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n; j++) {
if (temp[i][j] != g2[i][j]) {
f = 1;
break;
}
}
if (f == 1) break;
}
if (f == 0) cout << "两图同构^-^" << endl;
} while (next_permutation(a + 1, a + 1 + n));
cout << "两图不同构!!!" << endl;
}
return 0;
}
```
阅读全文
相关推荐
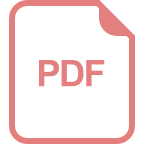











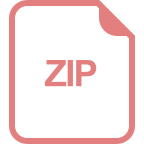
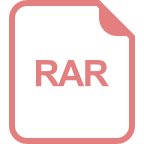
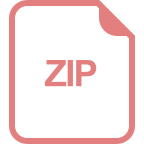