python编写国际象棋
时间: 2023-06-25 13:06:30 浏览: 166
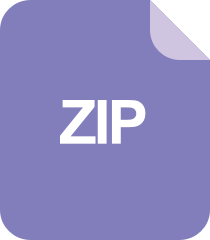
基于python的国际象棋.zip

编写国际象棋需要涉及到很多的规则和算法,需要考虑到棋子的移动、吃子、胜负判断等等。下面是一个简单的国际象棋游戏的代码示例:
```python
class Chessboard:
def __init__(self):
self.board = [['R', 'N', 'B', 'Q', 'K', 'B', 'N', 'R'],
['P', 'P', 'P', 'P', 'P', 'P', 'P', 'P'],
[' ', '.', ' ', '.', ' ', '.', ' ', '.'],
['.', ' ', '.', ' ', '.', ' ', '.', ' '],
[' ', '.', ' ', '.', ' ', '.', ' ', '.'],
['.', ' ', '.', ' ', '.', ' ', '.', ' '],
['p', 'p', 'p', 'p', 'p', 'p', 'p', 'p'],
['r', 'n', 'b', 'q', 'k', 'b', 'n', 'r']]
self.current_player = 'white'
def move(self, x1, y1, x2, y2):
piece = self.board[y1][x1]
if piece == ' ' or piece == '.':
return False
if (piece.isupper() and self.current_player == 'black') or \
(piece.islower() and self.current_player == 'white'):
return False
if not self.valid_move(x1, y1, x2, y2):
return False
self.board[y2][x2] = piece
self.board[y1][x1] = ' '
self.current_player = 'black' if self.current_player == 'white' else 'white'
return True
def valid_move(self, x1, y1, x2, y2):
piece = self.board[y1][x1]
dest_piece = self.board[y2][x2]
if dest_piece != ' ' and (piece.isupper() == dest_piece.isupper()):
return False
if piece.upper() == 'P':
if x1 == x2:
if dest_piece == ' ':
if y1 - y2 == 1:
return True
elif y1 == 6 and y2 == 4:
return True
return False
elif abs(x2 - x1) == 1 and y1 - y2 == 1 and dest_piece != ' ':
return True
else:
return False
# TODO: implement valid moves for other pieces
return True
```
这个示例只实现了棋子的移动和胜负判断。如果想要完整的国际象棋游戏,需要实现更多的规则和算法。
阅读全文
相关推荐
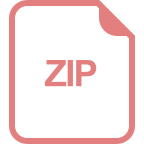
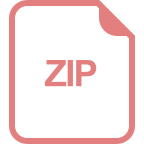

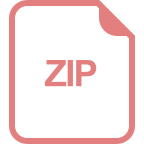
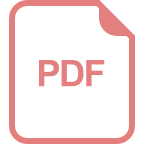
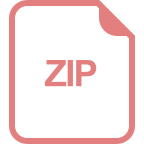
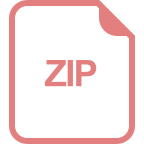
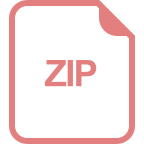
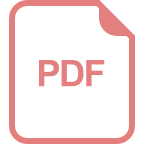
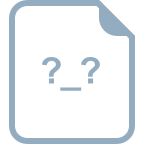
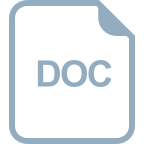
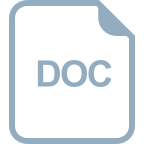
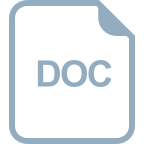
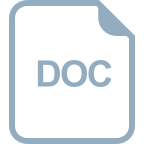
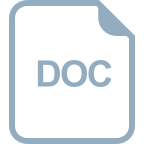
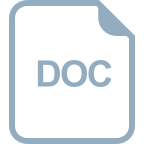

