HTML写一个静态网页(1) 图书管理系统首页设计 (2)图书管理系统注册页面设计使用CSS样式,JavaScript的使用
时间: 2023-09-22 21:06:44 浏览: 134
好的,以下是针对你提出的问题的代码示例和说明:
1. 图书管理系统首页设计
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>图书管理系统首页</title>
<style>
/* 导航栏样式 */
nav {
background-color: #333;
color: #fff;
height: 50px;
line-height: 50px;
text-align: center;
}
nav a {
color: #fff;
text-decoration: none;
margin: 0 20px;
}
/* 轮播图样式 */
.banner {
width: 100%;
height: 400px;
position: relative;
}
.banner img {
width: 100%;
height: 100%;
}
.banner .prev, .banner .next {
position: absolute;
top: 50%;
transform: translateY(-50%);
font-size: 30px;
color: #fff;
cursor: pointer;
}
.banner .prev {
left: 20px;
}
.banner .next {
right: 20px;
}
/* 图书分类样式 */
.category {
margin-top: 30px;
text-align: center;
}
.category h2 {
font-size: 24px;
margin-bottom: 20px;
}
.category ul {
list-style: none;
padding: 0;
margin: 0;
display: flex;
justify-content: center;
}
.category li {
margin: 0 10px;
}
.category a {
color: #333;
font-size: 16px;
text-decoration: none;
padding: 5px 10px;
border-radius: 5px;
border: 1px solid #ccc;
transition: all 0.2s ease;
}
.category a:hover {
background-color: #ccc;
border-color: #ccc;
color: #fff;
}
/* 推荐书籍样式 */
.recommend {
margin-top: 50px;
text-align: center;
}
.recommend h2 {
font-size: 24px;
margin-bottom: 20px;
}
.recommend ul {
list-style: none;
padding: 0;
margin: 0;
display: flex;
flex-wrap: wrap;
justify-content: center;
}
.recommend li {
margin: 20px;
box-shadow: 0 0 10px rgba(0,0,0,0.2);
border-radius: 5px;
overflow: hidden;
width: 200px;
height: 300px;
}
.recommend img {
width: 100%;
height: 200px;
object-fit: cover;
}
.recommend a {
display: block;
font-size: 16px;
color: #333;
text-decoration: none;
padding: 10px;
transition: all 0.2s ease;
}
.recommend a:hover {
background-color: #ccc;
color: #fff;
}
</style>
</head>
<body>
<!-- 导航栏 -->
<nav>
<a href="#">首页</a>
<a href="#">图书分类</a>
<a href="#">我的书架</a>
<a href="#">登录</a>
<a href="#">注册</a>
</nav>
<!-- 轮播图 -->
<div class="banner">
<img src="https://picsum.photos/1200/400" alt="轮播图1">
<img src="https://picsum.photos/1200/400?random=1" alt="轮播图2">
<img src="https://picsum.photos/1200/400?random=2" alt="轮播图3">
<div class="prev"><</div>
<div class="next">></div>
</div>
<!-- 图书分类 -->
<div class="category">
<h2>图书分类</h2>
<ul>
<li><a href="#">文学</a></li>
<li><a href="#">历史</a></li>
<li><a href="#">哲学</a></li>
<li><a href="#">科学</a></li>
<li><a href="#">艺术</a></li>
<li><a href="#">小说</a></li>
</ul>
</div>
<!-- 推荐书籍 -->
<div class="recommend">
<h2>推荐书籍</h2>
<ul>
<li>
<img src="https://picsum.photos/200/300" alt="书籍1">
<a href="#">书籍1</a>
</li>
<li>
<img src="https://picsum.photos/200/300?random=1" alt="书籍2">
<a href="#">书籍2</a>
</li>
<li>
<img src="https://picsum.photos/200/300?random=2" alt="书籍3">
<a href="#">书籍3</a>
</li>
<li>
<img src="https://picsum.photos/200/300?random=3" alt="书籍4">
<a href="#">书籍4</a>
</li>
<li>
<img src="https://picsum.photos/200/300?random=4" alt="书籍5">
<a href="#">书籍5</a>
</li>
<li>
<img src="https://picsum.photos/200/300?random=5" alt="书籍6">
<a href="#">书籍6</a>
</li>
</ul>
</div>
<script>
var banner = document.querySelector('.banner');
var prev = document.querySelector('.prev');
var next = document.querySelector('.next');
var index = 0;
var len = banner.children.length;
function show(index) {
for (var i = 0; i < len; i++) {
banner.children[i].style.display = 'none';
}
banner.children[index].style.display = 'block';
}
show(index);
prev.onclick = function() {
index--;
if (index < 0) {
index = len - 1;
}
show(index);
}
next.onclick = function() {
index++;
if (index >= len) {
index = 0;
}
show(index);
}
</script>
</body>
</html>
```
2. 图书管理系统注册页面设计
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>图书管理系统注册页面</title>
<style>
body {
background-color: #f7f7f7;
font-family: "Microsoft YaHei", sans-serif;
}
.container {
margin: 100px auto;
width: 500px;
background-color: #fff;
padding: 50px;
box-shadow: 0 0 10px rgba(0,0,0,0.2);
border-radius: 5px;
}
h1 {
font-size: 24px;
margin-bottom: 30px;
text-align: center;
}
form {
display: flex;
flex-direction: column;
align-items: center;
}
label {
font-size: 16px;
margin-bottom: 10px;
display: inline-block;
width: 100%;
}
input[type=text], input[type=password] {
width: 100%;
height: 40px;
padding: 5px;
font-size: 16px;
border-radius: 5px;
border: 1px solid #ccc;
margin-bottom: 20px;
box-sizing: border-box;
}
button {
background-color: #333;
color: #fff;
border-radius: 5px;
border: none;
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
transition: all 0.2s ease;
}
button:hover {
background-color: #fff;
color: #333;
border: 1px solid #333;
}
</style>
</head>
<body>
<div class="container">
<h1>注册</h1>
<form>
<label for="username">用户名</label>
<input type="text" id="username" name="username">
<label for="password">密码</label>
<input type="password" id="password" name="password">
<label for="confirm-password">确认密码</label>
<input type="password" id="confirm-password" name="confirm-password">
<button type="submit">注册</button>
</form>
</div>
<script>
var form = document.querySelector('form');
var username = document.querySelector('#username');
var password = document.querySelector('#password');
var confirm_password = document.querySelector('#confirm-password');
form.onsubmit = function() {
if (username.value.trim() === '') {
alert('用户名不能为空');
return false;
}
if (password.value.trim() === '') {
alert('密码不能为空');
return false;
}
if (password.value !== confirm_password.value) {
alert('两次输入的密码不一致');
return false;
}
}
</script>
</body>
</html>
```
以上是我提供的代码示例和说明,希望能够帮到你。如果你还有其他问题,可以随时问我。
阅读全文
相关推荐
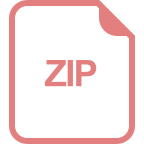
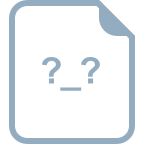



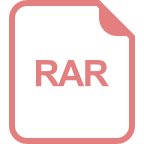
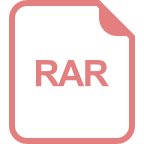
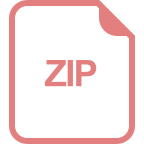
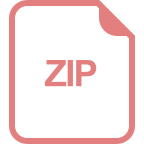
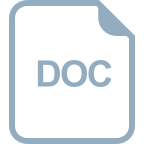
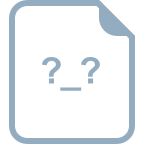
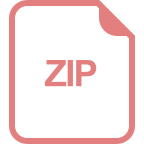
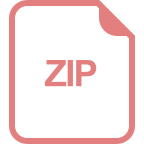
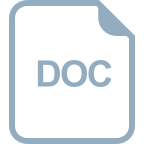
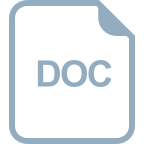
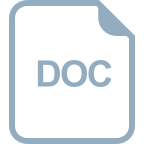
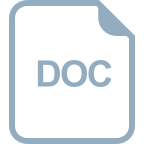
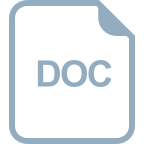