添加>重载
时间: 2023-08-10 09:04:38 浏览: 37
好的,以下是添加了 `>` 运算符重载的代码:
```c++
#include <iostream>
#include <cstring>
class MyString {
public:
MyString() : ptr(nullptr), len(0) {}
MyString(const char* str) {
len = std::strlen(str);
ptr = new char[len + 1];
std::strcpy(ptr, str);
}
MyString(const MyString& other) {
len = other.len;
ptr = new char[len + 1];
std::strcpy(ptr, other.ptr);
}
~MyString() {
if (ptr != nullptr) {
delete[] ptr;
ptr = nullptr;
}
}
MyString& operator=(const MyString& rhs) {
if (this != &rhs) {
if (ptr != nullptr) {
delete[] ptr;
}
len = rhs.len;
ptr = new char[len + 1];
std::strcpy(ptr, rhs.ptr);
}
return *this;
}
MyString operator+(const MyString& rhs) const {
MyString result;
result.len = len + rhs.len;
result.ptr = new char[result.len + 1];
std::strcpy(result.ptr, ptr);
std::strcat(result.ptr, rhs.ptr);
return result;
}
MyString& operator+=(const MyString& rhs) {
len += rhs.len;
char* temp = new char[len + 1];
std::strcpy(temp, ptr);
std::strcat(temp, rhs.ptr);
delete[] ptr;
ptr = temp;
return *this;
}
char& operator[](int index) {
return ptr[index];
}
void insert(const char* str, int pos) {
int str_len = std::strlen(str);
char* temp = new char[len + str_len + 1];
std::strncpy(temp, ptr, pos);
std::strcpy(temp + pos, str);
std::strcpy(temp + pos + str_len, ptr + pos);
delete[] ptr;
ptr = temp;
len += str_len;
}
bool operator>(const MyString& rhs) const {
return std::strcmp(ptr, rhs.ptr) > 0;
}
friend std::ostream& operator<<(std::ostream& os, const MyString& str);
private:
char* ptr;
int len;
};
std::ostream& operator<<(std::ostream& os, const MyString& str) {
os << str.ptr;
return os;
}
int main() {
MyString s1 = "Hello";
MyString s2 = "World";
std::cout << s1 << std::endl;
std::cout << s2 << std::endl;
s1 += s2;
std::cout << s1 << std::endl;
s1.insert(" ", 5);
std::cout << s1 << std::endl;
std::cout << s1[11] << std::endl;
std::cout << (s1 > s2) << std::endl;
return 0;
}
```
在这里,我们重载了 `>` 运算符,使得两个字符串对象可以进行比较,如果左边的字符串对象大于右边的字符串对象,则返回 `true`,否则返回 `false`。在 `operator>` 中,我们使用了 `strcmp` 函数来比较两个字符串的字典序大小。
相关推荐
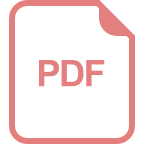
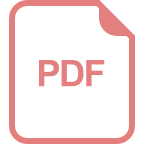














