mysql插入数据库时报错not all arguments converted during string formatting
时间: 2024-01-03 13:05:47 浏览: 166
当在MySQL插入数据库时出现"not all arguments converted during string formatting"错误时,这通常是由于插入语句中的占位符与提供的参数数量不匹配导致的。请确保插入语句中的占位符数量与提供的参数数量一致。
以下是一个示例,演示了如何使用占位符进行MySQL插入操作:
```python
import mysql.connector
# 连接到MySQL数据库
cnx = mysql.connector.connect(user='username', password='password',
host='localhost', database='database_name')
# 创建游标对象
cursor = cnx.cursor()
# 准备插入语句
insert_stmt = "INSERT INTO table_name (column1, column2) VALUES (%s, %s)"
# 提供要插入的参数
data = ('value1', 'value2')
# 执行插入操作
cursor.execute(insert_stmt, data)
# 提交更改
cnx.commit()
# 关闭游标和连接
cursor.close()
cnx.close()
```
请确保在执行插入操作时,提供的参数与占位符的数量和顺序一致。如果仍然出现错误,请检查插入语句和提供的参数是否正确。
相关问题
mysql insert data failed: not all arguments converted during string formatting
在MySQL中,当插入数据时出现"not all arguments converted during string formatting"错误通常是由于参数不正确导致的。这个错误可能有以下几种原因:
1. 参数数量不匹配:确保你提供的参数数量与表中定义的列数相匹配。
2. 参数顺序不正确:确认你按照正确的顺序提供了参数,以便与表中的列对应。
3. 数据类型不匹配:检查你提供的参数是否与表中定义的列的数据类型相匹配。如果不匹配,可以尝试进行适当的转换或更改数据类型。
4. 缺少必需的参数:确保你提供了所有必需的参数,并且没有遗漏任何一个。
下面是一个示例代码,展示了如何使用SQLAlchemy库来插入数据到MySQL数据库中:
```python
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.declarative import declarative_base
# 创建连接引擎
engine = create_engine('mysql+pymysql://username:password@localhost/database_name')
# 创建会话
Session = sessionmaker(bind=engine)
session = Session()
# 创建基类
Base = declarative_base()
# 定义模型类
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String(50))
age = Column(Integer)
# 创建数据对象
user = User(name='John', age=25)
# 添加数据对象到会话
session.add(user)
# 提交会话
session.commit()
# 关闭会话
session.close()
```
请注意,你需要将`username`、`password`和`database_name`替换为你自己的MySQL数据库的凭据和名称。
not all arguments converted during string formatting
### 回答1:
这是 Python 程序编码中的一种错误。它表示在将值插入到字符串中时,有些变量没有正确地转换为字符串。这可能是由于在字符串格式化语句中指定了多个占位符,但没有为所有占位符提供相应的参数所导致的。如果你能提供具体的错误代码,我可以为您提供更详细的信息。
### 回答2:
"Not all arguments converted during string formatting"是Python中常见的错误信息之一,通常出现在字符串格式化时。
在Python中,我们可以使用“%”或者“.format()”方法来对字符串进行格式化。格式化字符串中含有“%s”、“%d”、“%f”等占位符,用于指定要插入的变量的类型。如果字符串中的占位符与实际插入的变量类型不符,就会出现“not all arguments converted during string formatting”错误。
例如:
```
name = "xiaoming"
age = 18
height = 175.5
print("My name is %s, I'm %d years old and %.2f meters tall." % (name, age, height, weight))
```
上面的代码中,由于格式化字符串中只有三个占位符,而我们实际传入了四个变量,导致了“not all arguments converted during string formatting”错误。
为了解决这个问题,我们需要检查一下格式化字符串中的占位符数量是否与传入的变量数量相等。如果不相等,可以考虑修改格式化字符串或者添加相应的变量。
除了占位符数量的问题外,还有可能出现变量类型与占位符不匹配的情况。比如,当我们传入一个字符串类型的对象到%d的占位符中,就会发生“not all arguments converted during string formatting”错误。
总之,“not all arguments converted during string formatting”错误需要我们仔细检查格式化字符串和传入的变量,确保它们类型和数量的匹配。
### 回答3:
“not all arguments converted during string formatting”是Python中常见的错误,其主要原因是字符串格式化时缺少需要的参数。在Python中,字符串格式化通常使用百分号(%)进行操作,可以通过以下方式实现:
```
name = 'Tom'
age = 20
print('My name is %s and I am %d years old.' % (name, age))
```
在这个例子中,“%s”表示字符串格式,会被变量name的值替换,“%d”表示数字格式,会被变量age的值替换。注意字符串格式化中参数的数量、顺序和类型都需要与格式化字符串中的相应位置对应。
而当参数数量不足或参数类型不匹配时,就会出现“not all arguments converted during string formatting”错误。例如:
```
name = 'Tom'
age = 20
print('My name is %s.' % name, 'I am %d years old.' % age, 'My gender is %s.' % 'M')
```
在这个例子中,第二个字符串格式化的时候使用了“%d”占位符,但是没有传入任何数字参数,导致错误的产生。解决这个问题的方式是添加足够的参数,或者使用不同的格式化方式(例如f-string和format函数)来避免这种错误的发生。
总之,“not all arguments converted during string formatting”错误发生的原因是参数数量、类型不匹配或者缺少参数。在字符串格式化时,要注意参数的数量、顺序和类型的匹配,以避免这个错误的发生。
阅读全文
相关推荐
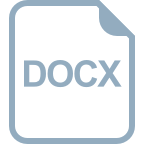
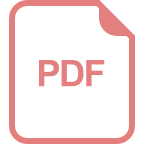




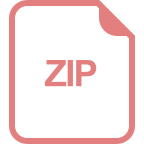
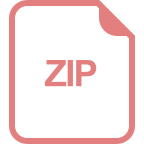
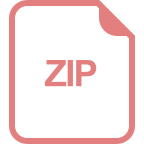
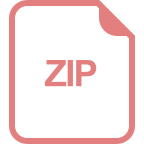