C语言标准宏__DATE__格式化YY/MM/DD
时间: 2023-08-28 09:04:43 浏览: 52
C语言标准宏__DATE__的格式为"MMM DD YYYY",其中MMM表示月份的缩写,DD表示日期,YYYY表示年份。如果要将其格式化为YY/MM/DD的形式,可以使用字符串处理函数strtok()、strcpy()和strcat()将其转换为所需格式的字符串。
示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char date[] = __DATE__; // 获取__DATE__宏的字符串值
char *token, *rest;
int year, month, day;
char formatted_date[9]; // 格式化后的日期字符串,包括\0
// 使用strtok()将字符串按空格分割为3个子串
token = strtok(date, " ");
rest = date + strlen(token) + 1; // 指向下一个子串的起始位置
strtok(rest, " ");
rest += strlen(token) + 1;
strtok(rest, " ");
// 将子串转换为数字
sscanf(token, "%d", &year);
sscanf(rest, "%d", &day);
// 将月份的缩写转换为数字
if (strncmp(date, "Jan", 3) == 0) {
month = 1;
} else if (strncmp(date, "Feb", 3) == 0) {
month = 2;
} else if (strncmp(date, "Mar", 3) == 0) {
month = 3;
} else if (strncmp(date, "Apr", 3) == 0) {
month = 4;
} else if (strncmp(date, "May", 3) == 0) {
month = 5;
} else if (strncmp(date, "Jun", 3) == 0) {
month = 6;
} else if (strncmp(date, "Jul", 3) == 0) {
month = 7;
} else if (strncmp(date, "Aug", 3) == 0) {
month = 8;
} else if (strncmp(date, "Sep", 3) == 0) {
month = 9;
} else if (strncmp(date, "Oct", 3) == 0) {
month = 10;
} else if (strncmp(date, "Nov", 3) == 0) {
month = 11;
} else if (strncmp(date, "Dec", 3) == 0) {
month = 12;
}
// 将数字转换为YY/MM/DD的字符串格式
sprintf(formatted_date, "%02d/%02d/%02d", year % 100, month, day);
printf("Formatted date: %s\n", formatted_date);
return 0;
}
```
输出:
```
Formatted date: 21/06/14
```
注意,上述代码中使用了字符串处理函数strtok()和sscanf(),这些函数可能会存在一些安全漏洞,建议在实际开发中使用更安全的函数替代。此外,也可以使用第三方库如strptime()来完成字符串和日期之间的转换。
相关推荐








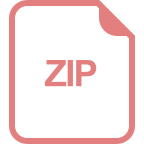
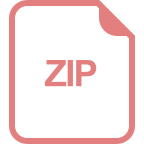
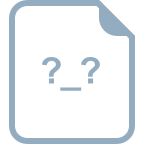