java群发模板消息
时间: 2023-11-21 14:58:50 浏览: 88
根据提供的引用内容,可以了解到Java群发模板消息需要进行以下步骤:
1. 开通微信公众号模板消息能力,具体操作可以参考引用中提供的微信文档。
2. 根据添加的消息模板和文档里面所需的参数设计表结构。
3. 编写Java代码实现模板消息的发送。
以下是Java代码实现模板消息发送的示例:
```java
// 导入相关的类库
import java.util.HashMap;
import java.util.Map;import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class WechatTemplateMessageUtil {
// 发送模板消息的接口地址
private static final String SEND_TEMPLATE_MESSAGE_URL = "https://api.weixin.qq.com/cgi-bin/message/template/send?access_token=ACCESS_TOKEN";
/**
* 发送模板消息
* @param accessToken 微信公众号的access_token
* @param openId 用户的openid
* @param templateId 模板消息的id
* @param data 模板消息的数据
* @return 发送结果
*/
public static String sendTemplateMessage(String accessToken, String openId, String templateId, Map<String, Object> data) {
// 构造请求参数
Map<String, Object> params = new HashMap<>();
params.put("touser", openId);
params.put("template_id", templateId);
params.put("data", data);
// 发送POST请求
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(SEND_TEMPLATE_MESSAGE_URL.replace("ACCESS_TOKEN", accessToken));
CloseableHttpResponse response = null;
String result = null;
try {
StringEntity entity = new StringEntity(JsonUtil.toJson(params), "UTF-8");
httpPost.setEntity(entity);
response = httpClient.execute(httpPost);
result = EntityUtils.toString(response.getEntity(), "UTF-8");
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (response != null) {
response.close();
}
httpClient.close();
} catch (Exception e) {
e.printStackTrace();
}
}
return result;
}
}
```
以上代码中,需要注意的是:
1. 需要导入相关的类库,例如Apache HttpClient和FastJson。
2. 需要替换SEND_TEMPLATE_MESSAGE_URL中的ACCESS_TOKEN为实际的access_token。
3. 需要传入openId、templateId和data等参数,其中data是一个Map类型的数据,包含了模板消息中需要替换的变量和对应的值。
阅读全文
相关推荐




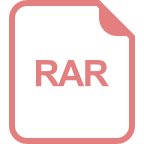
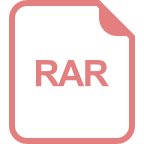


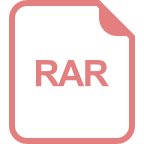
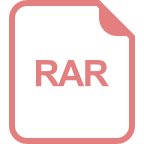

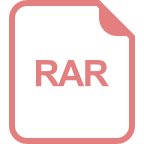



