matlab中利用C语言对图像进行“红”、“绿”、“蓝”分层提取,并将三幅分层图显示在一个窗口里,同时在这三个分层图的顶部附上图像的图名
时间: 2024-09-27 20:12:50 浏览: 55
在MATLAB中,你可以通过 Mex文件(MATLAB C/C++扩展)来调用C语言编写的功能,包括图像处理。对于RGB图像的分层提取,可以按照以下步骤操作:
1. **C语言部分**:
- 首先,你需要在C语言中读取图像数据并分别获取红色、绿色和蓝色分量。这通常涉及到遍历每个像素的RGB值。
```c
#include "mex.h"
#include <opencv2/opencv.hpp>
mxArray *extract_channel(const mxArray* image)
{
cv::Mat img = cv::matFromMexArray(image);
int height = img.rows;
int width = img.cols;
mxArray* red = mxCreateDoubleMatrix(height, width, mxREAL);
mxArray* green = mxCreateDoubleMatrix(height, width, mxREAL);
mxArray* blue = mxCreateDoubleMatrix(height, width, mxREAL);
for (int i = 0; i < height; ++i) {
for (int j = 0; j < width; ++j) {
cv::Vec3b pixel = img.at<cv::Vec3b>(i, j);
double r = static_cast<double>(pixel[0]);
double g = static_cast<double>(pixel[1]);
double b = static_cast<double>(pixel[2]);
mxSetPr(red, &r, j, 1);
mxSetPr(green, &g, j, 1);
mxSetPr(blue, &b, j, 1);
}
}
return std::vector<mxArray*>({red, green, blue});
}
```
2. **MATLAB部分**:
- 编写MEX函数来调用上述C函数,然后创建一个新的figure显示三张图片:
```matlab
function [img_red, img_green, img_blue] = cExtractRGBChannel(image)
[result, flag] = mex('extract_channel', image);
if ~flag
error('Error in C extraction');
end
img_red = result{1};
img_green = result{2};
img_blue = result{3};
figure;
subplot(1, 3, 1), imshow(img_red, []);
title('Red Channel');
subplot(1, 3, 2), imshow(img_green, []);
title('Green Channel');
subplot(1, 3, 3), imshow(img_blue, []);
title('Blue Channel');
end
```
3. **调用**:
- 将RGB图像传递给`cExtractRGBChannel`函数,它会返回并显示三个分层图像:
```matlab
im = imread('your_image.jpg'); % 替换为实际的图像文件路径
[img_red, img_green, img_blue] = cExtractRGBChannel(im);
```
关于你的问题,相关点可能会有:
阅读全文
相关推荐
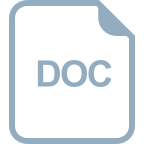
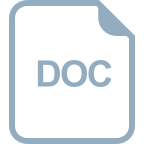
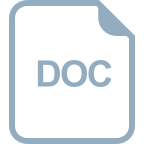
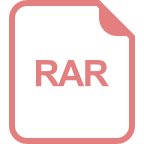
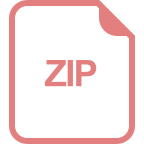
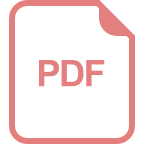
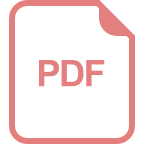
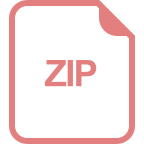
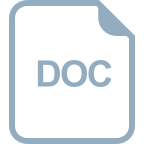
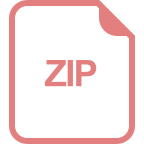
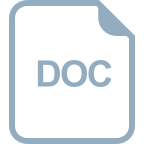
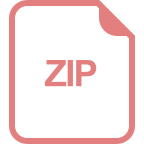
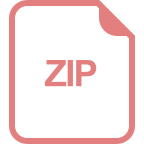
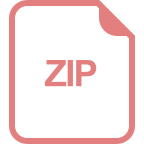
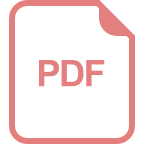
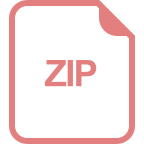
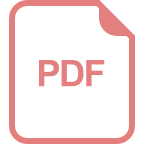
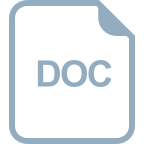
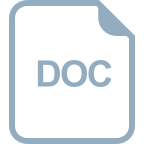
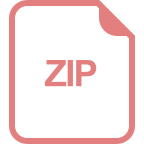