wpf如何实现框选元素
时间: 2024-04-19 14:28:17 浏览: 91
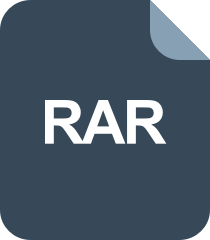
用WPF实现多选下拉框

要实现WPF中的框选元素,你可以使用鼠标事件和适当的布局面板。下面是一种常见的实现方式:
1. 在XAML中创建一个包含需要框选的元素的布局面板,例如Grid或Canvas。
2. 添加鼠标事件处理程序。在Grid或Canvas上订阅MouseLeftButtonDown、MouseLeftButtonUp和MouseMove事件。
3. 在MouseLeftButtonDown事件处理程序中,记录下鼠标按下的位置作为起始点。
4. 在MouseMove事件处理程序中,获取当前鼠标位置,并计算出框选区域的大小和位置。可以使用Mouse.GetPosition方法获取鼠标相对于Grid或Canvas的位置。
5. 更新框选区域的位置和大小。可以使用一个矩形元素或者一个透明的选择框元素来表示框选区域。
6. 在MouseLeftButtonUp事件处理程序中,完成框选操作。可以根据框选区域和元素的位置关系来确定被选中的元素。
以下是一个简单的示例,演示了如何在WPF中实现框选元素:
```xaml
<Grid MouseLeftButtonDown="OnMouseLeftButtonDown" MouseMove="OnMouseMove" MouseLeftButtonUp="OnMouseLeftButtonUp">
<Rectangle x:Name="SelectionBox" Stroke="Red" StrokeThickness="1" Fill="#80FF0000" Visibility="Collapsed"/>
<Rectangle Width="50" Height="50" Fill="Blue"/>
<Rectangle Width="50" Height="50" Fill="Green" Margin="70,0,0,0"/>
<Rectangle Width="50" Height="50" Fill="Yellow" Margin="140,0,0,0"/>
</Grid>
```
```csharp
private Point startPoint;
private void OnMouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
startPoint = e.GetPosition((UIElement)sender);
}
private void OnMouseMove(object sender, MouseEventArgs e)
{
if (e.LeftButton == MouseButtonState.Pressed)
{
Point currentPoint = e.GetPosition((UIElement)sender);
double minX = Math.Min(startPoint.X, currentPoint.X);
double minY = Math.Min(startPoint.Y, currentPoint.Y);
double maxX = Math.Max(startPoint.X, currentPoint.X);
double maxY = Math.Max(startPoint.Y, currentPoint.Y);
SelectionBox.Margin = new Thickness(minX, minY, 0, 0);
SelectionBox.Width = maxX - minX;
SelectionBox.Height = maxY - minY;
SelectionBox.Visibility = Visibility.Visible;
}
}
private void OnMouseLeftButtonUp(object sender, MouseButtonEventArgs e)
{
SelectionBox.Visibility = Visibility.Collapsed;
// 根据框选区域和元素位置判断被选中的元素
foreach (UIElement element in ((Grid)sender).Children)
{
if (element is Rectangle rect)
{
Rect elementRect = new Rect(element.TransformToAncestor((Grid)sender).Transform(new Point(0, 0)), new Size(rect.Width, rect.Height));
if (elementRect.IntersectsWith(new Rect(startPoint, e.GetPosition((UIElement)sender))))
{
// 处理被选中的元素
// ...
}
}
}
}
```
在这个示例中,我们使用一个Grid作为布局面板,并在其中添加了几个Rectangle元素作为可选元素。通过鼠标事件处理程序,我们可以实现框选元素的功能。在MouseLeftButtonUp事件处理程序中,我们可以根据框选区域和元素位置的关系来确定被选中的元素,并进行相应的处理。
希望对你有所帮助!
阅读全文
相关推荐
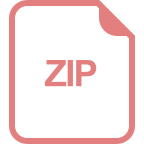
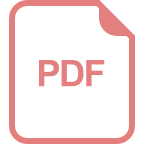
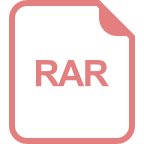
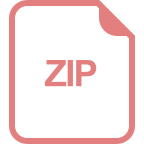
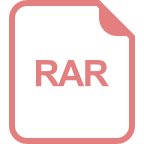
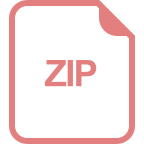
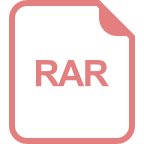
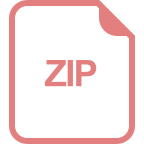
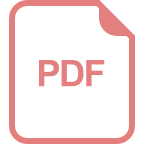
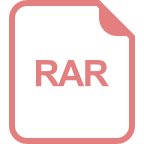
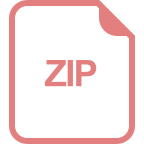
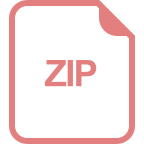
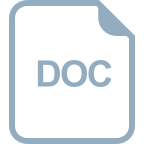
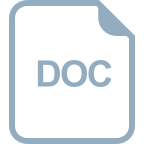
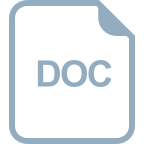
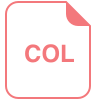