用matlab生成一个四人抢答器程序
时间: 2023-10-09 16:16:27 浏览: 226
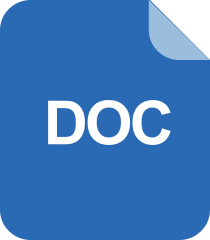
四人抢答器程序
好的,我可以为您提供一些思路和代码示例,帮助您实现一个基于 MATLAB 的四人抢答器程序。
首先,您需要定义四个按钮来代表四个玩家,同时还需要定义一个“开始游戏”按钮来启动抢答过程。当游戏开始时,每个玩家可以通过点击自己的按钮来回答问题。程序应该记录每个玩家的答题时间,并将最快的玩家作为本轮的胜者。当游戏结束时,程序应该显示本轮的胜者,并在下一轮开始前清空计时器和玩家答题状态。
接下来是一个简单的示例代码,用于创建四个按钮和一个“开始游戏”按钮,并记录每个玩家的答题时间:
```matlab
% 创建四个按钮和一个“开始游戏”按钮
player1Button = uicontrol('Style', 'pushbutton', 'String', 'Player 1', 'Position', [50 100 75 50]);
player2Button = uicontrol('Style', 'pushbutton', 'String', 'Player 2', 'Position', [150 100 75 50]);
player3Button = uicontrol('Style', 'pushbutton', 'String', 'Player 3', 'Position', [250 100 75 50]);
player4Button = uicontrol('Style', 'pushbutton', 'String', 'Player 4', 'Position', [350 100 75 50]);
startButton = uicontrol('Style', 'pushbutton', 'String', 'Start Game', 'Position', [200 200 100 50], 'Callback', @startGame);
% 定义全局变量来记录每个玩家的答题时间和状态
global player1Time player2Time player3Time player4Time;
global player1Answered player2Answered player3Answered player4Answered;
% 游戏开始时,将计时器和答题状态清零
function startGame(hObject, eventdata, handles)
player1Time = Inf;
player2Time = Inf;
player3Time = Inf;
player4Time = Inf;
player1Answered = false;
player2Answered = false;
player3Answered = false;
player4Answered = false;
end
% 玩家按下按钮后,记录答题时间和状态,并判断当前是否为本轮的胜者
function player1Callback(hObject, eventdata, handles)
if ~player1Answered
player1Time = tic;
player1Answered = true;
checkWinner();
end
end
function player2Callback(hObject, eventdata, handles)
if ~player2Answered
player2Time = tic;
player2Answered = true;
checkWinner();
end
end
function player3Callback(hObject, eventdata, handles)
if ~player3Answered
player3Time = tic;
player3Answered = true;
checkWinner();
end
end
function player4Callback(hObject, eventdata, handles)
if ~player4Answered
player4Time = tic;
player4Answered = true;
checkWinner();
end
end
% 检查当前是否有玩家已经回答,如果有则判断当前是否为本轮的胜者
function checkWinner()
if player1Answered && player2Answered && player3Answered && player4Answered
times = [toc(player1Time), toc(player2Time), toc(player3Time), toc(player4Time)];
[winTime, winner] = min(times);
fprintf('Winner is Player %d with time %f seconds.\n', winner, winTime);
player1Answered = false;
player2Answered = false;
player3Answered = false;
player4Answered = false;
end
end
% 为每个按钮添加回调函数
set(player1Button, 'Callback', @player1Callback);
set(player2Button, 'Callback', @player2Callback);
set(player3Button, 'Callback', @player3Callback);
set(player4Button, 'Callback', @player4Callback);
```
此示例代码仅实现了基本的四人抢答器功能,您可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
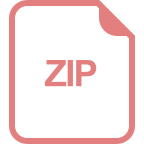
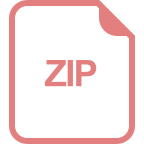
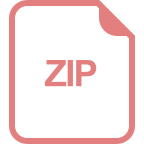
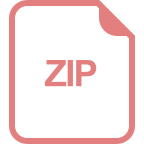
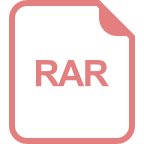
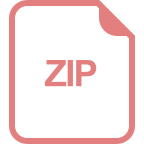
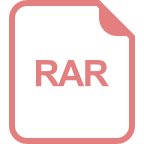
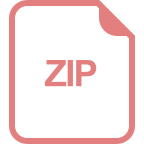
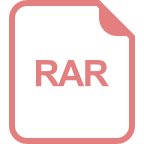
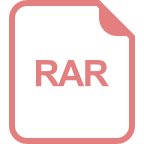
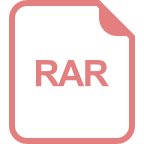
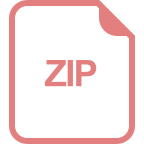