python输出box_python – 从tkinter.Listbox中提取项目列表
时间: 2024-02-27 20:59:16 浏览: 32
你可以使用Tkinter Listbox的curselection()方法获取选定项目的索引,然后使用get()方法提取项目列表。
以下是示例代码:
```
import tkinter as tk
def get_items():
selected_items = [listbox.get(idx) for idx in listbox.curselection()]
print(selected_items)
root = tk.Tk()
listbox = tk.Listbox(root, selectmode=tk.MULTIPLE)
listbox.pack()
listbox.insert(tk.END, "Item 1")
listbox.insert(tk.END, "Item 2")
listbox.insert(tk.END, "Item 3")
listbox.insert(tk.END, "Item 4")
listbox.insert(tk.END, "Item 5")
btn = tk.Button(root, text="Get selected items", command=get_items)
btn.pack()
root.mainloop()
```
此代码创建一个多选Listbox,并在其中添加5个项目。单击按钮后,将调用get_items()函数,该函数将使用curselection()和get()方法提取Listbox中所选项目的列表,并打印它们。
相关问题
用python的tkinter写一个GUI程序,包括菜单,一个按钮和两个显示框,按钮用来选择打开对话框选择文件,两个显示框,第1个用来显示打开的a.xlsl文件的第1个sheet的肉容,第2个显示框用来显示用鼠标选取的第1个显示框的行数据或列数据,直接给代码
使用Python的tkinter来创建一个GUI程序,包括菜单,一个按钮和两个显示框,具体的步骤如下:1. 导入tkinter模块:import tkinter2. 创建一个顶级窗口:top_window = tkinter.Tk()3. 创建一个菜单栏:menu_bar = tkinter.Menu(top_window)4. 创建一个按钮:button = tkinter.Button(top_window, text = "Open File", command = file_open)5. 创建两个显示框:display_box_1 = tkinter.Listbox(top_window)
display_box_2 = tkinter.Listbox(top_window)6. 将按钮和显示框添加到顶级窗口中:button.pack()
display_box_1.pack()
display_box_2.pack()7. 打开对话框选择文件:def file_open():
file_name = tkinter.filedialog.askopenfilename()8. 显示打开的a.xlsl文件的第1个sheet的内容:def display_file_content():
book = xlrd.open_workbook(file_name)
sheet = book.sheet_by_index(0)
for row in range(sheet.nrows):
for col in range(sheet.ncols):
display_box_1.insert(tkinter.END, sheet.cell_value(row, col))9. 显示用鼠标选取的第1个显示框的行数据或列数据:def display_row_or_col_data():
row = display_box_1.curselection()[0]
col = display_box_1.curselection()[1]
display_box_2.insert(tkinter.END, sheet.cell_value(row, col))
import tkinter as tk root=tk.Tk() root.title("Listbox+滚动条") #create a list box langs_var=tk.StringVar() listbox=tk.Listbox(root,listvariable=langs_var,selectmode='extended') for item in ['Java','C#','C','C++','Python','Go','JavaScript','PHP','Swift']: listbox.insert('end',item) for i in ['balana','apple','peach']: listbox.insert('end',i) listbox.pack() if listbox.curselection(): pass else: print("No selection") root.mainloop()
这是一个使用 tkinter 库创建 GUI 界面的 Python 代码,实现了一个带滚动条的列表框。具体解释如下:
1. 导入 tkinter 库,命名为 tk。
2. 创建一个根窗口对象,命名为 root。
3. 设置根窗口的标题为 "Listbox+滚动条"。
4. 创建一个变量对象 langs_var,用于存储列表框中的选项。
5. 创建一个列表框对象 listbox,将其绑定到根窗口,设置其显示模式为 'extended',并将其关联到变量 langs_var。
6. 使用 for 循环向列表框中添加选项。
7. 将列表框放置到根窗口中。
8. 判断列表框中是否有选中项,如果有则不执行任何操作,否则输出 "No selection"。
9. 进入主事件循环,等待用户操作。
需要注意的是,在判断列表框中是否有选中项时,应该将 if 语句放置在进入主事件循环之前,否则无法实时判断用户的选择。
相关推荐
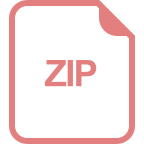








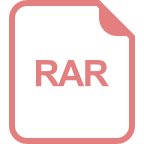