java 发起post请求
时间: 2024-10-25 16:00:43 浏览: 20
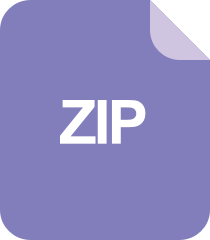
java发送http/https请求(get/post)Demo,亲测可用
在Java中,发起POST请求通常有两种常见的方式:使用基础的Java API `java.net.HttpURLConnection`,或者使用第三方库如Apache HttpClient或OkHttp。以下是使用`HttpURLConnection`的基本示例:
```java
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class PostRequest {
private static final String URL = "http://example.com/api"; // 替换为你实际的URL
private static final String PARAMS = "key1=value1&key2=value2"; // 示例参数
public void doPost() throws Exception {
URL url = new URL(URL);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 设置请求方法为POST
connection.setRequestMethod("POST");
// 添加请求头,告诉服务器这是个JSON请求
connection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
// 打开连接并获取输入流
OutputStream os = connection.getOutputStream();
os.write(PARAMS.getBytes()); // 写入参数
// 提交请求并关闭输入流
os.flush();
os.close();
// 获取响应码
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println("POST request successful");
// 读取并处理响应内容
byte[] responseData = readResponse(connection.getInputStream());
// 省略了响应数据的解析部分...
} else {
throw new RuntimeException("POST request failed with response code: " + responseCode);
}
// 关闭连接
connection.disconnect();
}
// 辅助函数用于从InputStream读取字节数组
private byte[] readResponse(InputStream in) throws Exception {
byte[] buffer = new byte[1024];
int length = 0;
byte[] data = new byte[length];
while ((length = in.read(buffer)) != -1) {
data = Arrays.copyOf(data, length + data.length);
System.arraycopy(buffer, 0, data, length, buffer.length);
}
return data;
}
}
```
如果你想使用更现代、功能丰富的库,如OkHttp,可以参考其文档来创建异步或线程安全的POST请求。
阅读全文
相关推荐
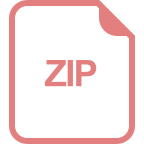


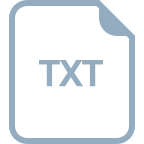
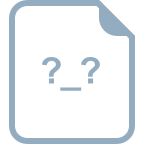
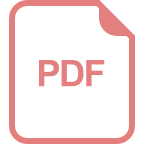
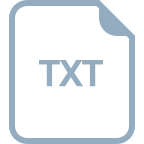
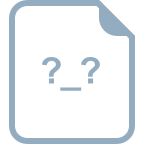
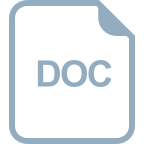
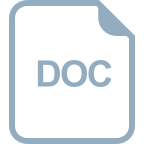




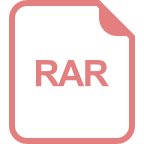

