编写一个程序,使之接收三个运行参数,分别为两个文件路径和一个无符号整数型,首先读取第一个路径对应文件内容,其中包含保存的若干用户名和明文密码,用无符号整数型作为偏移量对密码进行凯撒密码加密,将用户名和加密后的密码输出到第二个路径对应的文件中。
时间: 2024-02-22 11:59:25 浏览: 13
好的,这个问题需要用到文件操作和凯撒密码加密算法,我会为您提供一个 Python 的实现。
首先,我们需要导入 sys 模块来获取运行参数,使用 open 函数打开第一个文件,读取其中的用户名和明文密码,并对密码进行凯撒密码加密。然后将用户名和加密后的密码写入到第二个文件中。
以下是示例代码:
```python
import sys
def caesar_cipher(plaintext, shift):
"""
凯撒密码加密算法
"""
ciphertext = ""
for char in plaintext:
if char.isalpha():
char_code = (ord(char) - ord('a') + shift) % 26 + ord('a')
ciphertext += chr(char_code)
else:
ciphertext += char
return ciphertext
if len(sys.argv) != 4:
print("需要提供三个参数:第一个文件路径,第二个文件路径,整数型偏移量")
sys.exit(1)
input_file_path = sys.argv[1]
output_file_path = sys.argv[2]
shift = int(sys.argv[3])
with open(input_file_path, "r") as input_file, open(output_file_path, "w") as output_file:
for line in input_file:
username, password = line.strip().split(":")
encrypted_password = caesar_cipher(password, shift)
output_file.write(f"{username}:{encrypted_password}\n")
print("加密完成!")
```
您可以通过在命令行中运行以下命令来使用这个程序:
```
python3 encrypt.py input.txt output.txt 3
```
其中 `input.txt` 是第一个文件的路径,`output.txt` 是第二个文件的路径,`3` 是整数型偏移量。您可以根据需要替换这些参数。
相关推荐
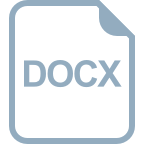
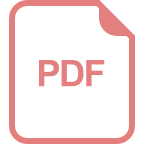














