ffmpeg视频加字幕
时间: 2023-07-17 20:07:08 浏览: 248
你可以使用FFmpeg来给视频添加字幕。下面是一个基本的命令示例:
```shell
ffmpeg -i input.mp4 -vf "subtitles=subtitles.srt" output.mp4
```
这个命令将`input.mp4`视频文件和`subtitles.srt`字幕文件合并,并输出为`output.mp4`。
请确保你已经安装了FFmpeg并将其添加到系统的环境变量中。字幕文件的格式应该是SRT格式。
你可以根据需要调整命令中的输入文件、输出文件和字幕文件的名称。还可以通过调整其他参数来进行更多的自定义,例如调整字幕的位置、字体、颜色等。你可以参考FFmpeg的官方文档以获取更多详细信息。
相关问题
易语言ffmpeg加字幕
易语言ffmpeg加字幕,可以通过以下步骤实现:
1.下载并安装ffmpeg:在官网上下载相应版本的ffmpeg,安装到本地电脑上。
2.准备好需要加字幕的视频文件和字幕文件。
3.打开易语言的IDE,在代码中引入FFMPEG的库文件,以及相关的其他库文件。
4.编写代码实现添加字幕的功能,主要是使用FFMPEG中的“-i”参数指定输入文件,使用“-vf”参数指定需要添加的字幕文件,以及“-c:v”和“-c:a”参数指定输出视频的编码格式和音频编码格式等等。
以下是一个简单的示例代码:
```
#include "e:\ffmpeg\include\libavcodec\avcodec.h"
#include "e:\ffmpeg\include\libavformat\avformat.h"
#include "e:\ffmpeg\include\libavfilter\avfilter.h"
#include "e:\ffmpeg\include\libswscale\swscale.h"
int main(int argc, char* argv[])
{
av_register_all();
avformat_network_init();
AVFormatContext *pFormatCtx = NULL;
int videoindex=-1;
AVCodecContext *pCodecCtxOrig=NULL;
AVCodecContext *pCodecCtx=NULL;
AVCodec *pCodec=NULL;
AVDictionary *optionsDict=NULL;
AVPacket *packet;
int ret, got_picture;
char filepath[]="test.mp4";
int i;
if(avformat_open_input(&pFormatCtx,filepath,NULL,NULL)!=0){
printf("Couldn't open input stream.\n");
return -1;
}
if(avformat_find_stream_info(pFormatCtx,NULL)<0){
printf("Couldn't find stream information.\n");
return -1;
}
for(i=0; i<pFormatCtx->nb_streams; i++)
if(pFormatCtx->streams[i]->codec->codec_type==AVMEDIA_TYPE_VIDEO){
videoindex=i;
break;
}
if(videoindex==-1){
printf("Didn't find a video stream.\n");
return -1;
}
pCodecCtxOrig=pFormatCtx->streams[videoindex]->codec;
pCodec=avcodec_find_decoder(pCodecCtxOrig->codec_id);
if(!pCodec){
printf("Codec not found.\n");
return -1;
}
pCodecCtx = avcodec_alloc_context3(pCodec);
if(avcodec_copy_context(pCodecCtx, pCodecCtxOrig) != 0) {
printf("Couldn't copy codec context.\n");
return -1; // Error copying codec context
}
if(avcodec_open2(pCodecCtx, pCodec, &optionsDict)<0){
printf("Could not open codec.\n");
return -1;
}
packet=(AVPacket *)av_malloc(sizeof(AVPacket));
AVFormatContext *ofmt_ctx = NULL;
avformat_alloc_output_context2(&ofmt_ctx, NULL, NULL, "output.mp4");
if (!ofmt_ctx) {
printf("Could not create output context\n");
return -1;
}
AVStream *out_stream = avformat_new_stream(ofmt_ctx, NULL);
if (!out_stream) {
printf("Failed allocating output stream\n");
return -1;
}
out_stream->codec->codec_tag = 0;
if (ofmt_ctx->oformat->flags & AVFMT_GLOBALHEADER)
out_stream->codec->flags |= AV_CODEC_FLAG_GLOBAL_HEADER;
avio_open(&ofmt_ctx->pb, "output.mp4", AVIO_FLAG_WRITE);
avformat_write_header(ofmt_ctx, NULL);
int got_frame=0;
AVFrame *pFrame = av_frame_alloc();
while(1){
if(av_read_frame(pFormatCtx, packet)>=0){
if(packet->stream_index==videoindex){
ret = avcodec_decode_video2(pCodecCtx, pFrame, &got_picture, packet);
if(ret < 0){
printf("Decode Error.\n");
return -1;
}
if(got_picture){
pFrame->pts=i++;
ret = avcodec_encode_video2(out_stream->codec, &out_pkt,pFrame, &got_frame);
if(ret < 0){
printf("Encode Error.\n");
return -1;
}
if (got_frame) {
out_pkt.stream_index = out_stream->index;
av_interleaved_write_frame(ofmt_ctx, &out_pkt);
av_free_packet(&out_pkt);
}
}
}
av_free_packet(packet);
}else
break;
}
}
//添加字幕
char cmd[100]={0};
sprintf(cmd,"ffmpeg -i test.mp4 -vf subtitles=subtitle.srt -c:v copy -c:a copy output.mp4");
system(cmd);
av_write_trailer(ofmt_ctx);
if (videoindex!=-1)
avcodec_close(pCodecCtx);
avformat_close_input(&pFormatCtx);
avformat_free_context(ofmt_ctx);
return 0;
}
java 视频加字幕_视频流添加文本合成字幕
您好,要实现视频流添加文本合成字幕的功能,可以使用 Java 编程语言结合使用一些开源库来实现。下面是一个简单的实现步骤:
1. 使用 FFmpeg 库将视频流转换为图片序列。
2. 使用 OpenCV 库读取每个图像,并在图像上添加字幕文本。
3. 将添加完字幕的图像序列再次使用 FFmpeg 库合并成视频流。
具体实现步骤如下:
1. 首先,需要在 Java 代码中使用 FFmpeg 库来将视频流转换成图像序列。可以使用 Jaffree 这个 Java API 来调用 FFmpeg 库。
2. 然后,使用 OpenCV 库来读取每个图像。对于每个图像,可以使用 OpenCV 的 putText 函数来添加字幕文本。putText 函数的参数包括要添加的文本、文本位置、字体、字号、颜色等。
3. 最后,将添加完字幕的图像序列再次使用 FFmpeg 库来合并成视频流。可以使用 Jaffree API 来调用 FFmpeg 库。
需要注意的是,这个实现过程还涉及到一些细节问题,如图像格式的转换、字幕的位置和样式设计等。如果您需要更详细的实现方法,可以参考一些相关的开源项目或者咨询专业人士。
阅读全文
相关推荐
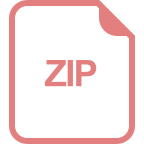
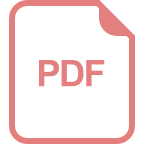
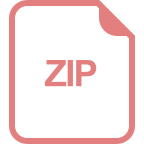
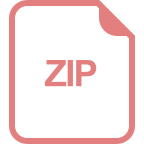
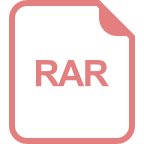
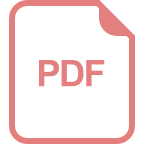
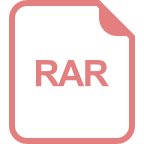
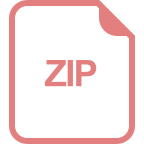
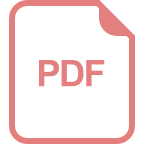
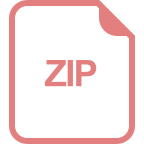
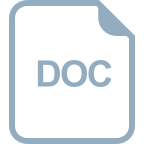
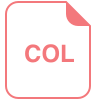
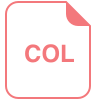
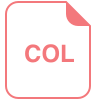
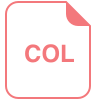