中断实现LED数码管计数显示,通过外部中断实现LED数码管计数显示。要求两个外部中断分别控制十位,个位数码管,从零开始,每按下1次,计数增加1,到9再变成0.写出来代码
时间: 2024-10-16 10:16:15 浏览: 10
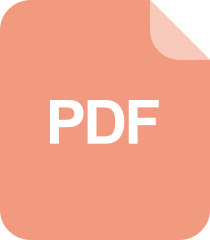
PLC实现LED数码管动态扫描数据显示
在嵌入式系统中,如使用Arduino Uno板,并利用INT0和INT1作为外部中断来控制LED数码管计数,你可以使用C语言编写这样的代码。这里是一个基本的示例,假设你已连接了数码管和外部中断按钮:
```c++
#include <LiquidCrystal.h> // Include the LiquidCrystal library for LCD display
// Define constants for LCD pins and interrupt pins
#define LCD_RS 8
#define LCD_EN 7
#define LCD_D4 4
#define LCD_D5 5
#define LCD_D6 6
#define LCD_D7 3
LiquidCrystal lcd(LCD_RS, LCD_EN, LCD_D4, LCD_D5, LCD_D6, LCD_D7); // Initialize LCD display
const int digitA = 9; // Pin for Digit A of LED display
const int digitB = 10; // Pin for Digit B of LED display
int count = 0; // Variable to store counting value
bool isInt0Pressed = false;
bool isInt1Pressed = false;
void setup() {
lcd.begin(16, 2); // Initialize LCD with 16x2 characters
pinMode(digitA, OUTPUT);
pinMode(digitB, OUTPUT);
attachInterrupt(digitalPinToInterrupt(INT0), incrementCounter, RISING); // Attach INT0 interrupt for digitA
attachInterrupt(digitalPinToInterrupt(INT1), incrementCounterB, RISING); // Attach INT1 interrupt for digitB
}
void loop() {
if (isInt0Pressed || isInt1Pressed) { // If any button is pressed
clearDisplay(); // Clear the LCD
}
displayCount(); // Display the current count
}
void incrementCounter() {
isInt0Pressed = true; // Set flag as button is pressed
count++;
if (count == 10) {
count = 0; // Reset count when reaching 10
}
shiftDisplay(count / 10, digitA); // Shift digits to A for tens place
}
void incrementCounterB() {
isInt1Pressed = true; // Set flag as button is pressed
count++;
if (count == 10) {
count = 0; // Reset count when reaching 10
}
shiftDisplay(count % 10, digitB); // Shift digits to B for ones place
}
void clearDisplay() {
lcd.clear();
}
void displayCount() {
lcd.setCursor(0, 0);
lcd.print("Count: ");
lcd.setCursor(0, 1);
if (count >= 10) {
shiftDisplay(count / 10, digitA);
shiftDisplay(count % 10, digitB);
} else {
shiftDisplay(count, digitA);
}
}
void shiftDisplay(int num, int pin) {
digitalWrite(pin, LOW); // Turn off previous digit
delayMicroseconds(1); // Allow time for human eye perception
while (num > 0) {
digitalWrite(pin, HIGH); // Light up the appropriate segment for the number
num--;
}
}
```
请确保将INT0和INT1替换为你实际硬件对应的中断引脚,并调整数码管引脚以适应你的电路布局。运行此代码后,每当外部中断触发时,计数就会递增并在LCD上显示。
阅读全文
相关推荐
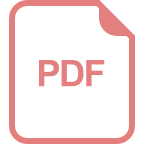
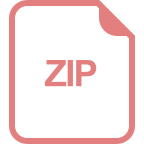
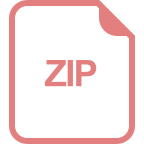
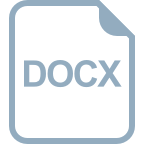
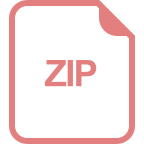
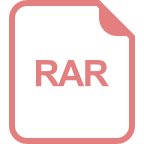
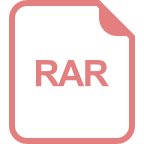
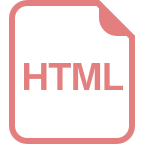
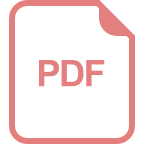
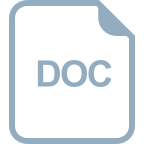
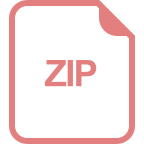
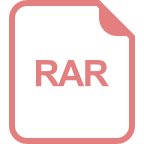
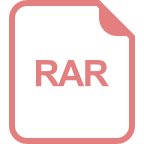
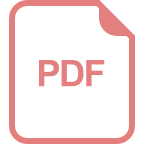
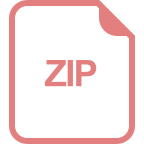
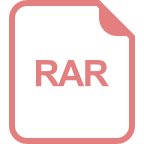