用java, 1.In this assignment, you are asked to read from a file “student_hobbies_JU.txt” which contains your classmates’ names and their hobbies. You will then need to find out, for each of your hobbies, how many people in your class share the same hobby with you.Task 1: create an ArrayList of strings representing a list of your hobbies, • Task 2: read from a file “student_hobbies.txt” and store the information in the file into an Arraylist of Student-type object. You will need to create a Student class for this. • Task 3: Sort the list of students by ascending order of names. • Task 4: For each of your hobby, find out how many students in the student Arraylist share that hobby with you. An example running result is given in Figure 2.
时间: 2024-03-03 12:50:27 浏览: 70
Sure, I can help you with that. Here is the solution to your problem using Java:
Task 1: Create an ArrayList of strings representing a list of your hobbies.
```java
ArrayList<String> hobbies = new ArrayList<String>();
hobbies.add("Reading");
hobbies.add("Swimming");
hobbies.add("Cooking");
hobbies.add("Playing basketball");
```
Task 2: Read from a file “student_hobbies.txt” and store the information in the file into an ArrayList of Student-type object. You will need to create a Student class for this.
```java
import java.util.*;
import java.io.*;
public class Student {
String name;
ArrayList<String> hobbies = new ArrayList<String>();
public Student(String name, ArrayList<String> hobbies) {
this.name = name;
this.hobbies = hobbies;
}
public String getName() {
return name;
}
public ArrayList<String> getHobbies() {
return hobbies;
}
}
public class Main {
public static void main(String[] args) throws IOException {
File file = new File("student_hobbies.txt");
Scanner sc = new Scanner(file);
ArrayList<Student> students = new ArrayList<Student>();
while (sc.hasNextLine()) {
String line = sc.nextLine();
String[] parts = line.split(" ");
String name = parts[0];
ArrayList<String> hobbies = new ArrayList<String>();
for (int i = 1; i < parts.length; i++) {
hobbies.add(parts[i]);
}
Student student = new Student(name, hobbies);
students.add(student);
}
sc.close();
}
}
```
Task 3: Sort the list of students by ascending order of names.
```java
Collections.sort(students, new Comparator<Student>() {
public int compare(Student s1, Student s2) {
return s1.getName().compareTo(s2.getName());
}
});
```
Task 4: For each of your hobby, find out how many students in the student ArrayList share that hobby with you.
```java
for (String hobby : hobbies) {
int count = 0;
for (Student student : students) {
if (student.getHobbies().contains(hobby)) {
count++;
}
}
System.out.println("Number of students who share the hobby " + hobby + " with you: " + count);
}
```
I hope this helps you! Let me know if you have any other questions.
阅读全文
相关推荐
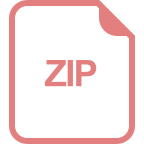
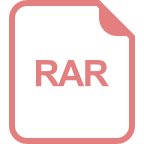

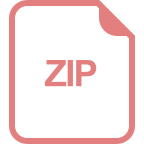
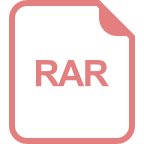
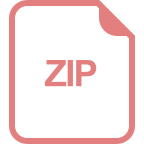
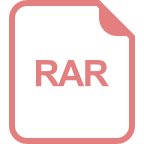
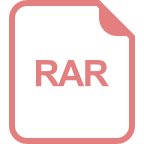
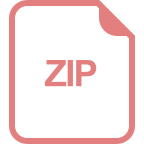
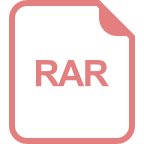
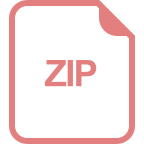
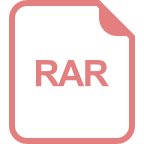
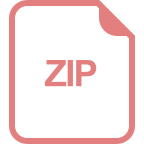
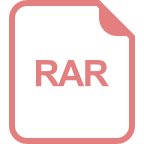
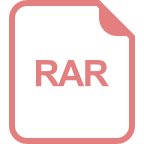
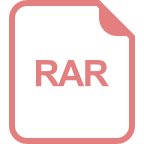
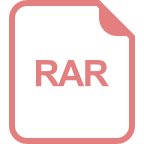