DES加密解密算法java实现代码密钥自己输入
时间: 2024-02-01 16:12:19 浏览: 111
下面是一个简单的DES加密解密算法的Java实现代码,密钥由用户输入:
```java
import javax.crypto.Cipher;
import javax.crypto.SecretKey;
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.DESKeySpec;
import java.util.Base64;
import java.util.Scanner;
public class DesDemo {
private static final String ALGORITHM = "DES";
public static void main(String[] args) throws Exception {
Scanner scanner = new Scanner(System.in);
// 获取密钥
System.out.print("请输入密钥:");
String keyString = scanner.nextLine();
byte[] keyBytes = keyString.getBytes();
// 生成密钥
DESKeySpec keySpec = new DESKeySpec(keyBytes);
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance(ALGORITHM);
SecretKey key = keyFactory.generateSecret(keySpec);
// 加密
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, key);
System.out.print("请输入要加密的字符串:");
String input = scanner.nextLine();
byte[] inputBytes = input.getBytes();
byte[] encryptedBytes = cipher.doFinal(inputBytes);
String encryptedString = Base64.getEncoder().encodeToString(encryptedBytes);
System.out.println("加密后的字符串:" + encryptedString);
// 解密
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(encryptedString));
String decryptedString = new String(decryptedBytes);
System.out.println("解密后的字符串:" + decryptedString);
}
}
```
运行该程序,会提示用户输入密钥和要加密的字符串,然后输出加密后的字符串和解密后的字符串。请注意,此处使用了Java的Base64编码和解码工具类,需要在Java 8及以上版本中使用。
阅读全文
相关推荐
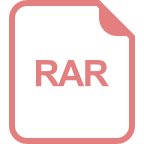
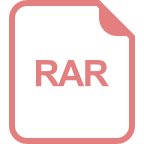
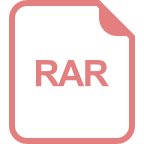
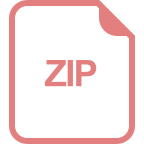
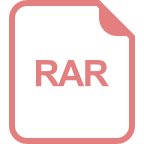
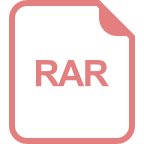
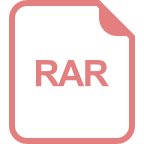
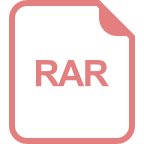
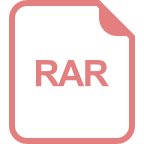
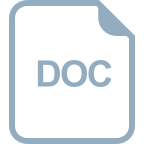
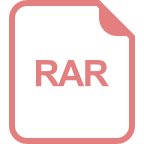
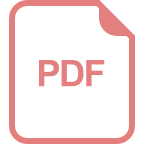
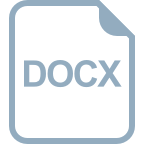
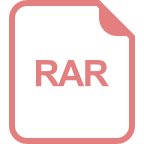