python使用BeautifulSoup定位某一个a标签,并进入页面
时间: 2024-06-08 11:07:06 浏览: 94
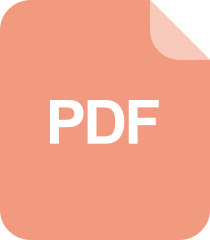
BeautifulSoup 获取 a标签里的文本内容
可以使用BeautifulSoup的find方法找到某一个a标签,然后获取它的href属性值,使用requests库访问这个链接即可进入页面。
示例代码如下:
```python
import requests
from bs4 import BeautifulSoup
# 访问页面并获取页面内容
url = 'https://www.example.com'
response = requests.get(url)
html = response.text
# 使用BeautifulSoup定位a标签
soup = BeautifulSoup(html, 'html.parser')
a_tag = soup.find('a', {'class': 'my-link'})
# 获取a标签的href属性值
link = a_tag['href']
# 访问链接
response = requests.get(link)
print(response.text)
```
上述代码中,我们首先使用requests库访问页面并获取页面内容。然后使用BeautifulSoup定位class为`my-link`的a标签,并获取它的href属性值。最后使用requests库访问这个链接并打印页面内容。
阅读全文
相关推荐
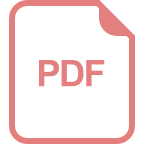
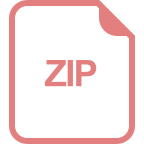


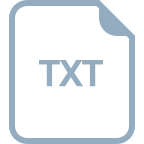
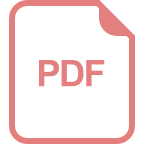
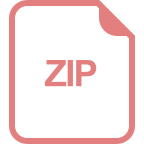
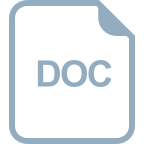
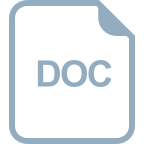
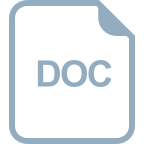
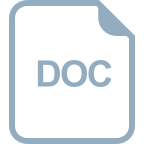
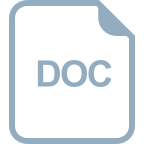
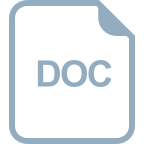
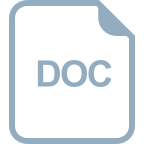
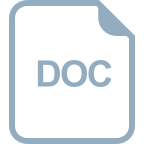
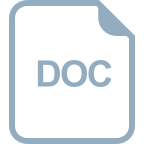
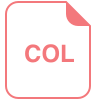