用python直接创建一个集合
时间: 2024-09-25 16:00:24 浏览: 7
在Python中,你可以使用内置的set()函数或者花括号 {} 直接创建一个集合。以下是两种常见的创建方法:
1. 使用 `set()` 函数:
```python
# 创建一个空集合
empty_set = set()
print(empty_set) # 输出: {}
# 创建包含元素的集合
filled_set = set([1, 2, 3, 4, 5])
print(filled_set) # 输出: {1, 2, 3, 4, 5}
```
2. 直接使用花括号:
```python
# 创建一个空集合
another_empty_set = {}
print(another_empty_set) # 输出: {}
# 同样可以添加元素
another_filled_set = {1, 2, 3, 4, 5}
print(another_filled_set) # 输出: {1, 2, 3, 4, 5}
```
注意,这里提到的`{}`实际上是字典的创建方式,但在Python 3.7及更高版本,如果里面只包含键值对会被视为集合。从字面意义上来讲,集合是一组无序、唯一的元素。
相关问题
python如何定义一个集合
在Python中,你可以使用内置的`set()`函数或者花括号`{}`来定义一个集合。集合是一组唯一的元素,没有重复项。下面是两个创建集合的方式:
1. 使用`set()`函数:
```python
# 定义一个空集合
empty_set = set()
# 或者从序列(如列表)创建集合
numbers = [1, 2, 3, 2, 1]
number_set = set(numbers)
```
2. 直接使用花括号:
```python
# 创建包含特定元素的集合
single_element_set = {42}
```
注意,集合的成员是无序的,而且集合不能有重复元素。
如何在Python中创建并操作集合?
在Python中,集合(Set)是一种无序、不重复元素的数据结构。你可以使用内置的`set()`函数或大括号`{}`来创建集合。以下是一些基本的操作:
1. 创建集合:
```python
# 使用 set() 函数
my_set = set([1, 2, 3, 4, 5])
# 直接用大括号
another_set = {6, 7, 8, 9, 10}
```
2. 添加元素:
```python
my_set.add(11) # 添加新的元素
```
3. 删除元素:
```python
my_set.remove(3) # 删除特定元素
```
4. 检查元素是否存在:
```python
if 2 in my_set:
print("2 is in the set")
```
5. 集合运算:
- 并集 (`|`):
```python
combined_set = my_set | another_set
```
- 交集 (`&`):
```python
intersection = my_set & another_set
```
- 差集 (`-`):
```python
difference = my_set - another_set
```
6. 集合大小:
```python
print(len(my_set))
```
7. 将列表转换为集合:
```python
list_to_convert = [1, 2, 3, 2, 1]
unique_list = list(set(list_to_convert))
```
8. 集合的迭代:
```python
for item in my_set:
print(item)
```
相关推荐
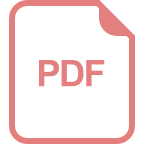
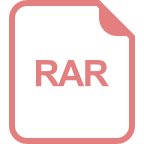
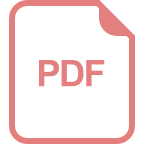












