Java如何实现跨服务器上传下载文件
时间: 2023-05-31 09:02:19 浏览: 132
Java可以使用以下两种方式实现跨服务器上传下载文件:
1. 使用FTP协议:FTP协议是一种文件传输协议,可以实现跨服务器上传下载文件。Java中可以使用Apache Commons Net库来实现FTP文件传输。示例代码如下:
```
import org.apache.commons.net.ftp.*;
public class FtpExample {
public static void main(String[] args) {
String server = "ftp.example.com";
int port = 21;
String user = "username";
String pass = "password";
FTPClient client = new FTPClient();
try {
client.connect(server, port);
client.login(user, pass);
client.enterLocalPassiveMode();
String remoteFile = "/path/to/remote/file";
File localFile = new File("/path/to/local/file");
OutputStream outputStream = new BufferedOutputStream(new FileOutputStream(localFile));
boolean success = client.retrieveFile(remoteFile, outputStream);
outputStream.close();
if (success) {
System.out.println("File downloaded successfully.");
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (client.isConnected()) {
client.logout();
client.disconnect();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
2. 使用HTTP协议:HTTP协议也可以用于文件传输,可以通过HTTP的POST和GET请求来实现文件上传和下载。Java中可以使用Apache HttpClient库来实现HTTP文件传输。示例代码如下:
```
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.FileBody;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class HttpExample {
public static void main(String[] args) {
HttpClient httpClient = HttpClients.createDefault();
// 文件上传
HttpPost httpPost = new HttpPost("http://example.com/upload");
FileBody fileBody = new FileBody(new File("/path/to/local/file"));
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addPart("file", fileBody);
httpPost.setEntity(builder.build());
try {
HttpResponse response = httpClient.execute(httpPost);
String result = EntityUtils.toString(response.getEntity());
System.out.println(result);
} catch (IOException e) {
e.printStackTrace();
}
// 文件下载
HttpGet httpGet = new HttpGet("http://example.com/download?file=/path/to/remote/file");
try {
HttpResponse response = httpClient.execute(httpGet);
HttpEntity entity = response.getEntity();
if (entity != null) {
InputStream inputStream = entity.getContent();
OutputStream outputStream = new BufferedOutputStream(new FileOutputStream("/path/to/local/file"));
byte[] buffer = new byte[1024];
int len = 0;
while ((len = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, len);
}
outputStream.close();
inputStream.close();
EntityUtils.consume(entity);
System.out.println("File downloaded successfully.");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐





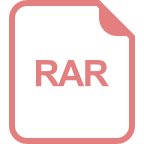





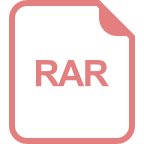
