分支限界法:布线问题Java代码
时间: 2024-02-26 19:56:48 浏览: 69
以下是使用分支限界法解决布线问题的Java代码示例:
```java
import java.util.*;
public class BranchAndBound {
private static int n; // 线段数
private static int[][] wires; // 线段的起点和终点坐标
private static int[] path; // 当前路径
private static int[] bestPath; // 最优路径
private static int minLen; // 当前最小长度
private static int[] used; // 记录线段是否已经使用
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
System.out.println("请输入线段的数量:");
n = scan.nextInt();
wires = new int[n][2];
path = new int[n];
bestPath = new int[n];
used = new int[n];
for (int i = 0; i < n; i++) {
System.out.println("请输入第" + (i + 1) + "条线段的起点和终点坐标:");
wires[i][0] = scan.nextInt();
wires[i][1] = scan.nextInt();
}
scan.close();
minLen = Integer.MAX_VALUE;
dfs(0, 0);
System.out.println("最短路径为:");
for (int i = 0; i < n; i++) {
System.out.print(bestPath[i] + " ");
}
System.out.println("\n最短路径长度为:" + minLen);
}
private static void dfs(int depth, int len) {
if (depth == n) {
if (len < minLen) {
minLen = len;
System.arraycopy(path, 0, bestPath, 0, n);
}
return;
}
for (int i = 0; i < n; i++) {
if (used[i] == 0) {
path[depth] = i;
used[i] = 1;
if (depth == 0) {
dfs(depth + 1, 0);
} else {
int curLen = len + getLen(wires[path[depth - 1]], wires[i]);
if (curLen < minLen) {
dfs(depth + 1, curLen);
}
}
used[i] = 0;
}
}
}
private static int getLen(int[] wire1, int[] wire2) {
return Math.abs(wire1[0] - wire2[0]) + Math.abs(wire1[1] - wire2[1]);
}
}
```
在上述代码中,我们使用了dfs进行搜索,每次尝试将一个线段加入当前路径,并计算路径长度,如果比当前最优解要小,则继续搜索。同时我们使用了一个used数组记录每一个线段是否已经被使用过,避免重复使用。最终输出找到的最短路径和路径长度。
阅读全文
相关推荐
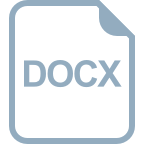
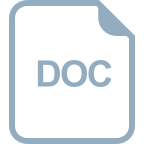
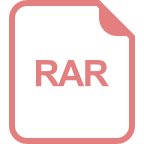
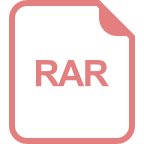
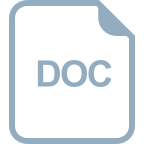
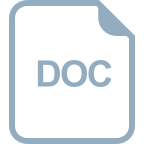
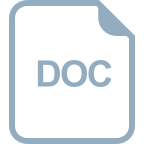
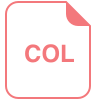
