请编写JAVA程序完成以下HDFS操作:(1)创建目录 (2)创建文件 (3) 读取文件内容 (4)文件的删除 5) 文件的上传 6)文件的下载 (7)列表显示指定目录下的文件和子目录信息(8) 复制文件 (9) 重命名文件
时间: 2023-12-05 11:03:43 浏览: 32
好的,下面是JAVA程序实现HDFS操作的代码:
1. 创建目录
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class CreateDirectory {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
Path dirPath = new Path("/testDir");
if (fs.mkdirs(dirPath)) {
System.out.println("Directory created successfully");
} else {
System.out.println("Failed to create directory");
}
}
}
```
2. 创建文件
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class CreateFile {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
Path filePath = new Path("/testDir/test.txt");
if (fs.createNewFile(filePath)) {
System.out.println("File created successfully");
} else {
System.out.println("Failed to create file");
}
}
}
```
3. 读取文件内容
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class ReadFile {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
Path filePath = new Path("/testDir/test.txt");
BufferedReader reader = new BufferedReader(new InputStreamReader(fs.open(filePath)));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
}
}
```
4. 文件的删除
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class DeleteFile {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
Path filePath = new Path("/testDir/test.txt");
if (fs.delete(filePath, false)) {
System.out.println("File deleted successfully");
} else {
System.out.println("Failed to delete file");
}
}
}
```
5. 文件的上传
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class UploadFile {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
Path srcPath = new Path("localFilePath");
Path dstPath = new Path("/hdfsFilePath");
fs.copyFromLocalFile(srcPath, dstPath);
System.out.println("File uploaded successfully");
}
}
```
6. 文件的下载
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class DownloadFile {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
Path srcPath = new Path("/hdfsFilePath");
Path dstPath = new Path("localFilePath");
fs.copyToLocalFile(srcPath, dstPath);
System.out.println("File downloaded successfully");
}
}
```
7. 列表显示指定目录下的文件和子目录信息
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileStatus;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class ListFiles {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
Path dirPath = new Path("/testDir");
FileStatus[] status = fs.listStatus(dirPath);
for (FileStatus fileStatus : status) {
if (fileStatus.isDirectory()) {
System.out.println("Directory: " + fileStatus.getPath());
} else if (fileStatus.isFile()) {
System.out.println("File: " + fileStatus.getPath());
}
}
}
}
```
8. 复制文件
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class CopyFile {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
Path srcPath = new Path("/srcPath");
Path dstPath = new Path("/dstPath");
fs.rename(srcPath, dstPath);
System.out.println("File copied successfully");
}
}
```
9. 重命名文件
```
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class RenameFile {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
Path oldPath = new Path("/oldPath");
Path newPath = new Path("/newPath");
fs.rename(oldPath, newPath);
System.out.println("File renamed successfully");
}
}
```
以上是可以完成HDFS操作的JAVA代码,请根据需要进行修改和使用。
相关推荐
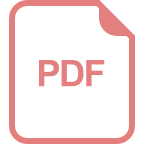
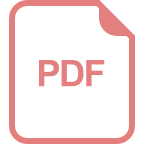













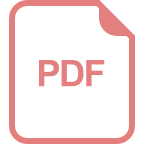