Java写一个租房管理系统,统计每个的电费金额
时间: 2024-11-16 17:20:29 浏览: 14
在Java中创建一个租房管理系统,我们首先需要设计一个基础的数据结构来存储租户信息以及他们的用电情况。例如,可以创建一个`Tenant`类,包含姓名、房间号和一个记录电表读数和对应费用的方法。然后,我们可以创建一个`ElectricityRecord`类来跟踪每次的读数变化。
下面是一个简单的示例:
```java
class ElectricityRecord {
private double previousReading;
private double currentReading;
public ElectricityRecord(double previousReading, double currentReading) {
this.previousReading = previousReading;
this.currentReading = currentReading;
}
public double calculateBill() {
if (previousReading <= 0 || currentReading <= 0) {
throw new IllegalArgumentException("Invalid reading");
}
double kilowattHours = (currentReading - previousReading) * electricityRate; // 假设有一个固定的电价electricityRate
return kilowattHours * unitPricePerKwh; // 单价unitPricePerKwh
}
private static final double electricityRate = 0.6; // 每度电的价格
private static final double unitPricePerKwh = 0.5; // 单价
}
class Tenant {
private String name;
private String roomNumber;
private List<ElectricityRecord> records;
public Tenant(String name, String roomNumber) {
this.name = name;
this.roomNumber = roomNumber;
this.records = new ArrayList<>();
}
public void addElectricityRecord(double previousReading, double currentReading) {
records.add(new ElectricityRecord(previousReading, currentReading));
}
public double getTotalElectricityBill() {
return records.stream().mapToDouble(ElectricityRecord::calculateBill).sum();
}
}
// 使用示例
public class RentManagementSystem {
public static void main(String[] args) {
Tenant tenant = new Tenant("张三", "A001");
tenant.addElectricityRecord(1000, 1200); // 假设单位为千瓦时
tenant.addElectricityRecord(1200, 1500);
double totalBill = tenant.getTotalElectricityBill();
System.out.printf("租户 %s 的电费总额是 %.2f 元\n", tenant.getName(), totalBill);
}
}
```
阅读全文
相关推荐
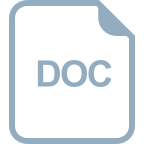
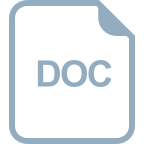
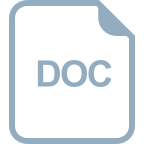
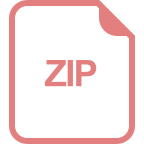
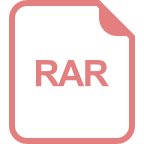
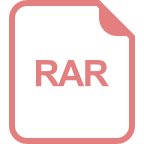
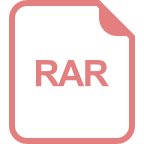
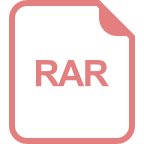
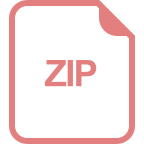
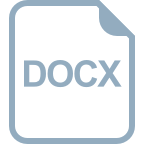
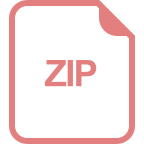
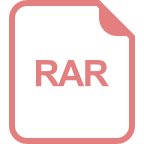
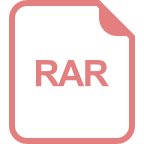
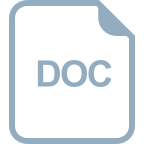
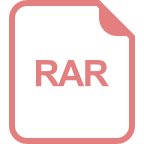
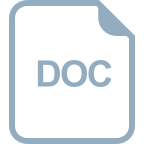