、题目一:采用 scikit-learn 中的线性 SVM 对 iris 数据集进行二分类。 具体内容: (1)选取两个特征和两类数据使用 scikit-learn 中的 SVM 进行二分类。 (2)输出:决策边界的参数和截距、支持向量等。 (3)可视化:通过散点图可视化数据样本(之前选择的两个特征),并画出决策边界和 2 个最 大间隔边界,标出支持向量用pychaem写
时间: 2024-02-16 08:00:01 浏览: 24
好的,根据你的要求,我们可以使用 scikit-learn 中的线性 SVM 模型对 iris 数据集进行二分类,并且输出决策边界的参数和截距、支持向量等。同时,我们还需要可视化数据样本和决策边界,并且标出支持向量。下面是完整代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import load_iris
from sklearn.svm import SVC
from sklearn.model_selection import train_test_split
# 加载 iris 数据集
iris = load_iris()
X = iris.data[:, :2] # 只选取前两个特征
y = iris.target
# 可视化数据集
plt.scatter(X[y == 0][:, 0], X[y == 0][:, 1], label='class 0')
plt.scatter(X[y == 1][:, 0], X[y == 1][:, 1], label='class 1')
plt.legend()
plt.show()
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# 建立线性 SVM 模型
clf = SVC(kernel='linear')
clf.fit(X_train, y_train)
# 输出决策函数的参数和截距,以及支持向量
print('theta:', clf.coef_)
print('intercept:', clf.intercept_)
print('support vectors:', clf.support_vectors_)
# 可视化决策边界和支持向量
plt.scatter(X[y == 0][:, 0], X[y == 0][:, 1], label='class 0')
plt.scatter(X[y == 1][:, 0], X[y == 1][:, 1], label='class 1')
plt.scatter(clf.support_vectors_[:, 0], clf.support_vectors_[:, 1], s=100, facecolors='none', edgecolors='k')
x_min, x_max = X[:, 0].min() - 0.5, X[:, 0].max() + 0.5
y_min, y_max = X[:, 1].min() - 0.5, X[:, 1].max() + 0.5
xx, yy = np.meshgrid(np.linspace(x_min, x_max, 100), np.linspace(y_min, y_max, 100))
Z = clf.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
plt.contour(xx, yy, Z, levels=[-1, 0, 1], linestyles=['--', '-', '--'])
plt.legend()
plt.show()
```
代码中,我们首先加载 iris 数据集,并且只选取前两个特征。然后可视化数据集,并且划分出训练集和测试集。接着,我们建立线性 SVM 模型,输出决策函数的参数和截距,以及支持向量。最后,我们可视化决策边界和支持向量,将二分类问题的边界可视化出来。
希望这个代码能够帮到你!
相关推荐
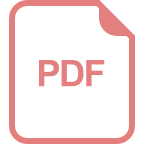
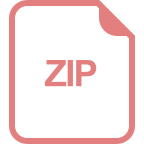
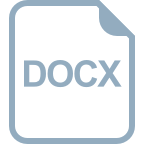













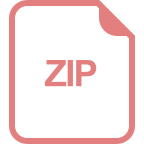