list<Book>lst; class Bookstore { private: list<Book> books; public: void WarehousingBook() {//入库书籍 cout << "请输入书名:"; string name; cin >> name; cout << "请输入作者:"; string author; cin >> author; cout << "请输入出版社:"; string publisher; cin >> publisher; cout << "请输入页数:"; int pages; cin >> pages; cout << "请输入最新入库时间(格式:年 月 日):"; int year, month, day; cin >> year >> month >> day; Time latestTime(year, month, day); cout << "请输入进货数量:"; int stock; cin >> stock; cout << "请输入价格:"; double price; cin >> price; list<Book>::iterator it; for (it = lst.begin(); it != lst.end(); it++){ if (it->name == name && it->author == author) { it->stock += stock; cout << "该书库里存在该书,入库成功!" << endl; return; } } Book b(name, author, publisher, pages, latestTime, stock, price); books.push_back(b); cout << "该书库里未存在该书,入库成功!" << endl; } 为什么实现不了
时间: 2024-01-15 22:02:55 浏览: 24
这段代码可能实现不了的原因是因为lst和books是两个不同的list对象,且在函数中只对lst进行了遍历和操作,而将新书籍添加到了books中。所以在函数结束后,books中的内容并没有被更新。如果想要实现添加新书籍的功能,需要将遍历和操作的对象改为books。同时,如果需要在多个函数中共享books这个list对象,需要将其定义为类的私有成员变量。修改后的代码如下所示:
```
class Bookstore {
private:
list<Book> books; // 书籍列表
public:
void WarehousingBook() { // 入库书籍
cout << "请输入书名:";
string name;
cin >> name;
cout << "请输入作者:";
string author;
cin >> author;
cout << "请输入出版社:";
string publisher;
cin >> publisher;
cout << "请输入页数:";
int pages;
cin >> pages;
cout << "请输入最新入库时间(格式:年 月 日):";
int year, month, day;
cin >> year >> month >> day;
Time latestTime(year, month, day);
cout << "请输入进货数量:";
int stock;
cin >> stock;
cout << "请输入价格:";
double price;
cin >> price;
list<Book>::iterator it;
for (it = books.begin(); it != books.end(); it++) {
if (it->name == name && it->author == author) {
it->stock += stock;
cout << "该书库里存在该书,入库成功!" << endl;
return;
}
}
Book b(name, author, publisher, pages, latestTime, stock, price);
books.push_back(b);
cout << "该书库里未存在该书,入库成功!" << endl;
}
};
```
相关推荐
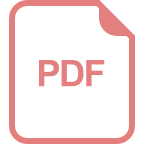
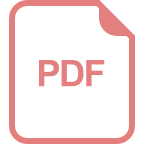
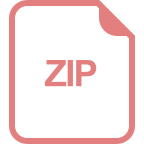














