java把yyyy/mm/dd变成yyyy-mm-dd
时间: 2023-03-29 17:03:59 浏览: 123
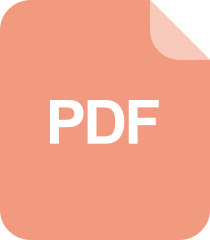
Javascript将字符串日期格式化为yyyy-mm-dd的方法

可以使用 SimpleDateFormat 类中的 format 方法将日期格式从 yyyy/mm/dd 转换为 yyyy-mm-dd。具体代码如下:
```
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateConverter {
public static void main(String[] args) {
String dateString = "2022/01/01";
SimpleDateFormat inputFormat = new SimpleDateFormat("yyyy/MM/dd");
SimpleDateFormat outputFormat = new SimpleDateFormat("yyyy-MM-dd");
try {
Date date = inputFormat.parse(dateString);
String outputDateString = outputFormat.format(date);
System.out.println(outputDateString);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
输出结果为:2022-01-01。
阅读全文
相关推荐
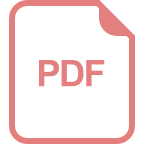















