std map triple
时间: 2023-09-05 08:09:37 浏览: 51
std::map is a container in C++ that stores elements in key-value pairs, where each key is unique. However, since you mentioned "triple," I assume you are referring to a map with a composite key consisting of three values. Unfortunately, std::map does not support composite keys out of the box.
To achieve a map with a triple key, you have a few options:
1. Use std::tuple as the key type: You can create a std::tuple of three values and use it as the key type in std::map. Here's an example:
```cpp
#include <iostream>
#include <map>
#include <tuple>
int main() {
std::map<std::tuple<int, int, int>, std::string> tripleMap;
tripleMap[std::make_tuple(1, 2, 3)] = "Value 1";
tripleMap[std::make_tuple(4, 5, 6)] = "Value 2";
std::cout << tripleMap[std::make_tuple(1, 2, 3)] << std::endl; // Output: Value 1
return 0;
}
```
2. Implement a custom key type: If you need more control over the key type or want to define custom comparison operations, you can create a struct or class to represent the triple and overload the necessary operators (e.g., operator<). Then, you can use this custom key type in std::map.
```cpp
#include <iostream>
#include <map>
struct Triple {
int x;
int y;
int z;
bool operator<(const Triple& other) const {
return std::tie(x, y, z) < std::tie(other.x, other.y, other.z);
}
};
int main() {
std::map<Triple, std::string> tripleMap;
tripleMap[{1, 2, 3}] = "Value 1";
tripleMap[{4, 5, 6}] = "Value 2";
std::cout << tripleMap[{1, 2, 3}] << std::endl; // Output: Value 1
return 0;
}
```
These are two possible approaches to handling a map with a triple key in C++. Choose the one that suits your needs and provides the desired functionality.
相关推荐
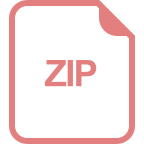














