写一个复杂的有地鼠图案,有背景图,而且界面居中的html打地鼠代码
时间: 2024-04-05 22:35:28 浏览: 11
以下是一个稍微复杂一些的打地鼠游戏代码,包含了地鼠图案和背景图,并将游戏界面居中显示:
```html
<!DOCTYPE html>
<html>
<head>
<title>打地鼠游戏</title>
<style type="text/css">
body {
background-image: url('https://cdn.pixabay.com/photo/2016/11/08/05/26/wallpaper-1801197_960_720.jpg');
background-size: cover;
background-position: center;
}
.container {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-template-rows: repeat(3, 1fr);
grid-gap: 10px;
margin: 0 auto;
margin-top: 50px;
width: 600px;
height: 600px;
}
.hole {
background-image: url('https://cdn.pixabay.com/photo/2014/03/24/13/57/mole-295329_960_720.png');
background-repeat: no-repeat;
background-size: contain;
width: 200px;
height: 200px;
display: flex;
justify-content: center;
align-items: center;
cursor: pointer;
transition: background-position 0.3s ease-in-out;
}
.hole:hover {
background-position: bottom;
}
.hole.active {
background-position: top;
}
</style>
</head>
<body>
<h1>打地鼠游戏</h1>
<div class="container">
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
<div class="hole"></div>
</div>
<script type="text/javascript">
const holes = document.querySelectorAll('.hole');
let score = 0;
let lastHole;
let timeUp = false;
function randomTime(min, max) {
return Math.round(Math.random() * (max - min) + min);
}
function randomHole(holes) {
const idx = Math.floor(Math.random() * holes.length);
const hole = holes[idx];
if (hole === lastHole) {
return randomHole(holes);
}
lastHole = hole;
return hole;
}
function peep() {
const time = randomTime(200, 1000);
const hole = randomHole(holes);
hole.classList.add('active');
setTimeout(() => {
hole.classList.remove('active');
if (!timeUp) {
peep();
}
}, time);
}
function startGame() {
score = 0;
timeUp = false;
document.getElementById('score').textContent = score;
peep();
setTimeout(() => {
timeUp = true;
}, 10000);
}
function bonk(e) {
if (!e.isTrusted) {
return; // cheater!
}
score++;
this.classList.remove('active');
document.getElementById('score').textContent = score;
}
holes.forEach(hole => hole.addEventListener('click', bonk));
</script>
<p>得分:<span id="score">0</span></p>
<button onclick="startGame()">开始游戏</button>
</body>
</html>
```
该代码使用了两张图片作为游戏背景和地鼠图案,并使用了 CSS 属性 `background-image` 和 `background-position` 来设置它们的位置和大小。游戏界面使用了网格布局,通过设置 `margin` 和 `width`、`height` 属性来居中显示。在 JavaScript 部分,相对于之前的代码稍作修改,用 `background-position` 属性替代了 `opacity` 属性来控制地鼠图案的显示状态。
相关推荐
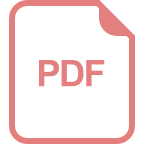
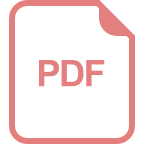














