Tensorflow 实现自定义层:改写常规模型中的任意模块或者层,并实现对mnist数据集进行图像分类,具体代码
时间: 2023-12-03 12:44:03 浏览: 35
好的,以下是一个使用 TensorFlow 实现自定义层的示例,包括对MNIST数据集进行图像分类的完整代码:
```python
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras.layers import Layer
# 自定义层
class CustomLayer(Layer):
def __init__(self, output_dim, **kwargs):
self.output_dim = output_dim
super(CustomLayer, self).__init__(**kwargs)
def build(self, input_shape):
self.kernel = self.add_weight(name='kernel',
shape=(input_shape[1], self.output_dim),
initializer='uniform',
trainable=True)
super(CustomLayer, self).build(input_shape)
def call(self, inputs):
return tf.matmul(inputs, self.kernel)
def compute_output_shape(self, input_shape):
return (input_shape[0], self.output_dim)
# 加载数据集
mnist = keras.datasets.mnist
(train_images, train_labels), (test_images, test_labels) = mnist.load_data()
# 数据预处理
train_images = train_images / 255.0
test_images = test_images / 255.0
# 构建模型
model = keras.Sequential([
keras.layers.Flatten(input_shape=(28, 28)),
CustomLayer(128),
keras.layers.Dense(10, activation=tf.nn.softmax)
])
# 编译模型
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(train_images, train_labels, epochs=5)
# 评估模型
test_loss, test_acc = model.evaluate(test_images, test_labels)
print('Test accuracy:', test_acc)
```
在上面的代码中,我们定义了一个名为 `CustomLayer` 的自定义层,它是 `Layer` 类的子类。这个自定义层接受一个参数 `output_dim`,表示输出维度,然后定义了一个 `build()` 方法和一个 `call()` 方法来实现层的功能。`build()` 方法用于初始化层的权重,在这里我们使用了一个随机均匀分布来初始化权重。`call()` 方法用于实现前向传播,这里我们使用了矩阵乘法来计算输出。
接下来,我们加载了 MNIST 数据集,对数据进行预处理,并构建了一个包含自定义层的模型。我们使用了 `compile()` 方法来编译模型,并使用 `fit()` 方法来训练模型。最后,我们使用 `evaluate()` 方法来评估模型的性能,输出测试集的准确率。
希望这个示例能够帮助你理解如何在 TensorFlow 中实现自定义层,并构建图像分类模型。
相关推荐
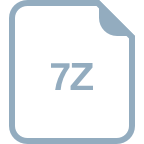
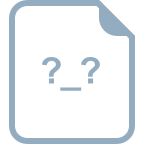
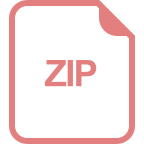














