使用c++,请通过重载函数,创建自己的 string 类,并命名为MyString, MyString 类是一个抽象数据类型,用来处理字符串,实现以下功能: 1)自动实现内存的动态分配,程序员无须关心为一个数组分配多少个字节的空间; 2)可以将string 对象直接赋值给MyString 对象,无须调用strcpy函数; 3)采用“+=”运算符可以连接两个 MyString 对象,无须使用 strcat 函数。 4)采用==、<、> 和!=运算符实现两个对象的比较,无须调用 strcmp 函数。
时间: 2024-03-11 11:50:41 浏览: 52
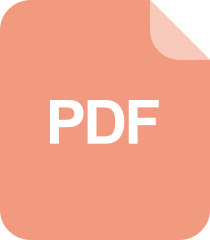
详解C++中实现继承string类的MyString类的步骤
好的,以下是一个简单的示例代码,实现了您所描述的 MyString 类的基本功能:
```c++
#include <iostream>
#include <cstring>
class MyString {
private:
char* str;
public:
MyString() : str(nullptr) {}
MyString(const char* s) {
if (s) {
str = new char[strlen(s) + 1];
strcpy(str, s);
} else {
str = nullptr;
}
}
MyString(const MyString& other) {
if (other.str) {
str = new char[strlen(other.str) + 1];
strcpy(str, other.str);
} else {
str = nullptr;
}
}
~MyString() {
delete[] str;
}
MyString& operator=(const char* s) {
if (str) {
delete[] str;
str = nullptr;
}
if (s) {
str = new char[strlen(s) + 1];
strcpy(str, s);
}
return *this;
}
MyString& operator=(const MyString& other) {
if (this != &other) {
if (str) {
delete[] str;
str = nullptr;
}
if (other.str) {
str = new char[strlen(other.str) + 1];
strcpy(str, other.str);
}
}
return *this;
}
MyString& operator+=(const MyString& other) {
if (other.str) {
char* temp = new char[strlen(str) + strlen(other.str) + 1];
strcpy(temp, str);
strcat(temp, other.str);
if (str) {
delete[] str;
}
str = temp;
}
return *this;
}
bool operator==(const MyString& other) const {
return strcmp(str, other.str) == 0;
}
bool operator<(const MyString& other) const {
return strcmp(str, other.str) < 0;
}
bool operator>(const MyString& other) const {
return strcmp(str, other.str) > 0;
}
bool operator!=(const MyString& other) const {
return strcmp(str, other.str) != 0;
}
friend std::ostream& operator<<(std::ostream& os, const MyString& obj) {
if (obj.str) {
os << obj.str;
}
return os;
}
};
int main() {
MyString s1("hello");
MyString s2("world");
MyString s3 = s1;
MyString s4 = "hello";
std::cout << s1 << std::endl; // 输出 hello
std::cout << (s1 == s2) << std::endl; // 输出 0
std::cout << (s1 < s2) << std::endl; // 输出 1
std::cout << (s1 > s2) << std::endl; // 输出 0
std::cout << (s1 != s2) << std::endl; // 输出 1
s1 += s2;
std::cout << s1 << std::endl; // 输出 helloworld
s1 = s2 = s3 = s4;
std::cout << s1 << std::endl; // 输出 hello
std::cout << s2 << std::endl; // 输出 hello
std::cout << s3 << std::endl; // 输出 hello
std::cout << s4 << std::endl; // 输出 hello
return 0;
}
```
在这个示例代码中,我们定义了一个 MyString 类,并实现了以下操作:
1. 构造函数和析构函数:使用 new 动态分配内存,自动实现内存的动态分配和释放;
2. 赋值运算符:重载了 = 运算符,可以直接将一个字符串或另一个 MyString 对象赋值给当前对象,无须调用 strcpy 函数;
3. += 运算符:重载了 += 运算符,可以直接将两个 MyString 对象连接起来,无须使用 strcat 函数;
4. 比较运算符:重载了 ==、<、> 和 != 运算符,可以直接比较两个 MyString 对象,无须调用 strcmp 函数;
5. 友元函数:重载了 << 运算符,使得可以直接使用 cout 输出 MyString 对象。
当然,这只是一个简单的示例代码,实际上 MyString 类还可以实现更多的操作,比如子串查找、替换、插入、删除等等。
阅读全文
相关推荐
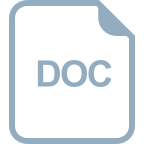
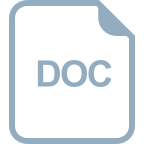
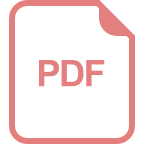
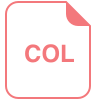
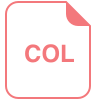
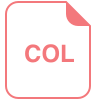
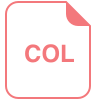
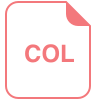
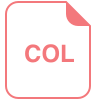
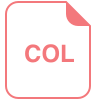
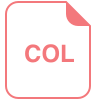
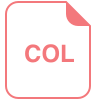
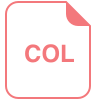
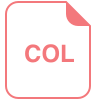
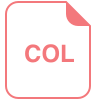
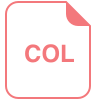
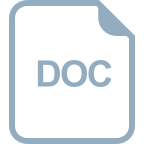