获取一个网页内超链接内的内容
时间: 2023-11-21 14:05:08 浏览: 91
要获取一个网页内超链接中的内容,可以使用 Python 的 requests 库和 BeautifulSoup 库来实现。具体步骤如下:
1. 使用 requests 库发送 HTTP 请求,获取网页的 HTML 内容。
2. 使用 BeautifulSoup 库解析 HTML 内容,查找所有的超链接标签(a 标签)。
3. 遍历所有的超链接标签,获取其 href 属性值,即链接地址。
4. 对于每个链接地址,使用 requests 库发送 HTTP 请求,获取链接页面的 HTML 内容。
5. 使用 BeautifulSoup 库解析链接页面的 HTML 内容,获取需要的内容。
下面是一个示例代码,它可以获取指定网页中所有超链接的标题和内容:
```python
import requests
from bs4 import BeautifulSoup
# 发送 HTTP 请求,获取网页内容
url = "https://example.com"
response = requests.get(url)
html = response.content
# 解析 HTML 内容,查找所有的超链接
soup = BeautifulSoup(html, "html.parser")
links = soup.find_all("a")
# 遍历每个超链接,获取链接页面的内容
for link in links:
href = link.get("href")
if href:
# 发送 HTTP 请求,获取链接页面的内容
link_response = requests.get(href)
link_html = link_response.content
# 解析链接页面的 HTML 内容,获取需要的内容
link_soup = BeautifulSoup(link_html, "html.parser")
title = link_soup.find("title").get_text()
content = link_soup.find("div", {"class": "content"}).get_text()
# 输出标题和内容
print("Title:", title)
print("Content:", content)
```
注意,这只是一个简单的示例代码,实际应用中可能需要根据具体的网页结构来进行调整。
阅读全文
相关推荐



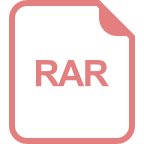













