python 生成一边录音一边显示波形的多线程程序
时间: 2023-10-05 21:06:38 浏览: 60
好的,我可以回答这个问题。您可以使用Python的pyaudio库来录制音频,并使用matplotlib库来显示波形。可以使用多线程来同时进行录制和显示。以下是一个简单的示例代码:
```python
import pyaudio
import matplotlib.pyplot as plt
import numpy as np
import threading
CHUNK = 1024
FORMAT = pyaudio.paInt16
CHANNELS = 1
RATE = 44100
frames = []
stop_flag = False
def record_audio():
global stop_flag, frames
p = pyaudio.PyAudio()
stream = p.open(format=FORMAT, channels=CHANNELS, rate=RATE,
input=True, frames_per_buffer=CHUNK)
while not stop_flag:
data = stream.read(CHUNK)
frames.append(data)
stream.stop_stream()
stream.close()
p.terminate()
def plot_waveform():
global stop_flag, frames
plt.ion()
fig = plt.figure()
wf = fig.add_subplot(111)
wf.set_ylim(-32768, 32768)
wf.set_xlim(0, CHUNK)
wf.set_xlabel('Time')
wf.set_ylabel('Amplitude')
plt.title('Live Audio Waveform')
while not stop_flag:
if len(frames) > 0:
data = frames.pop(0)
data_int = np.fromstring(data, 'int16')
wf.clear()
wf.plot(data_int)
plt.pause(0.0001)
plt.close()
t1 = threading.Thread(target=record_audio)
t1.start()
t2 = threading.Thread(target=plot_waveform)
t2.start()
input("Press Enter to stop recording")
stop_flag = True
t1.join()
t2.join()
```
这个程序使用两个线程,一个线程录制音频数据存储在 `frames` 列表中,另一个线程显示波形图。主线程在按下回车键后停止录制和显示。这是一个简单的多线程示例,您可以根据自己的需求调整程序。
相关推荐
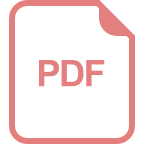
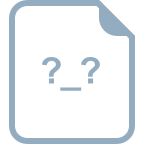
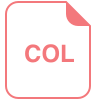
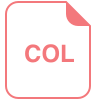
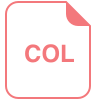











