python 重写calcHist
时间: 2024-02-18 20:37:37 浏览: 37
在 OpenCV 中,calcHist 函数用于计算图像的直方图。如果你想重写 calcHist 函数,你可以使用 Numpy 来实现。下面是一个简单的实现:
```python
import numpy as np
def calcHist(img, bins):
hist = np.zeros(bins, dtype=int)
for pixel in img.flat:
hist[pixel] += 1
return hist
```
这个函数接受一个图像数组和直方图的 bin 数量作为参数,并返回一个包含直方图值的数组。
这个函数的实现非常简单,它首先创建一个大小为 bins 的零数组,然后遍历图像中的每一个像素,并将相应的 bin 的计数器加一。最后,函数返回直方图数组。
请注意,在这个实现中,我们使用了 Numpy 的 flat 属性来将图像数组展平成一个一维数组。这样可以方便地遍历每个像素。
相关问题
用python重写calcHist
在OpenCV中,calcHist函数用于计算图像的直方图。以下是使用Python重新实现calcHist函数的示例代码:
```python
import numpy as np
def calcHist(images, channels, mask, histSize, ranges):
""" Compute the histogram of the input image(s).
:param images: input image(s) as a numpy array or list of numpy arrays.
:param channels: list of indices of channels to be used for histogram calculation.
:param mask: optional mask used to select pixels for histogram calculation.
:param histSize: list of bin counts for each histogram dimension.
:param ranges: list of ranges for each histogram dimension.
:return: computed histogram(s) as a numpy array or list of numpy arrays.
"""
# Check if images is a list of images or a single image
if isinstance(images, list):
# Compute histogram for each image in the list
hist_list = []
for image in images:
hist = calcHist(image, channels, mask, histSize, ranges)
hist_list.append(hist)
return hist_list
# Compute histogram for a single image
hist = np.zeros(histSize, dtype=np.float32)
dims = len(histSize)
# Convert ranges to numpy arrays
ranges = [np.asarray(r, dtype=np.float32) for r in ranges]
# Compute the bin widths
bin_widths = []
for r, size in zip(ranges, histSize):
bin_widths.append((r[1] - r[0]) / size)
# Compute the histogram
rows, cols = images.shape[:2]
if dims == 1:
for row in range(rows):
for col in range(cols):
if mask is None or mask[row, col]:
val = images[row, col, channels[0]]
if ranges[0][0] <= val < ranges[0][1]:
bin_index = int((val - ranges[0][0]) // bin_widths[0])
hist[bin_index] += 1
elif dims == 2:
for row in range(rows):
for col in range(cols):
if mask is None or mask[row, col]:
val1 = images[row, col, channels[0]]
val2 = images[row, col, channels[1]]
if ranges[0][0] <= val1 < ranges[0][1] and ranges[1][0] <= val2 < ranges[1][1]:
bin_index1 = int((val1 - ranges[0][0]) // bin_widths[0])
bin_index2 = int((val2 - ranges[1][0]) // bin_widths[1])
hist[bin_index1, bin_index2] += 1
elif dims == 3:
for row in range(rows):
for col in range(cols):
if mask is None or mask[row, col]:
val1 = images[row, col, channels[0]]
val2 = images[row, col, channels[1]]
val3 = images[row, col, channels[2]]
if ranges[0][0] <= val1 < ranges[0][1] and ranges[1][0] <= val2 < ranges[1][1] and ranges[2][0] <= val3 < ranges[2][1]:
bin_index1 = int((val1 - ranges[0][0]) // bin_widths[0])
bin_index2 = int((val2 - ranges[1][0]) // bin_widths[1])
bin_index3 = int((val3 - ranges[2][0]) // bin_widths[2])
hist[bin_index1, bin_index2, bin_index3] += 1
else:
raise ValueError("Images with more than 3 channels are not supported.")
return hist
```
这个函数有五个输入参数和一个输出参数:
- images: 输入图像,可以是一个numpy数组或者一个numpy数组的列表
- channels: 用来计算直方图的通道,是一个索引列表
- mask: 一个可选的掩膜,只有掩膜内的像素才会被用来计算直方图
- histSize: 直方图的bin数目,是一个整数列表
- ranges: 每个bin的范围,是一个浮点数列表的列表
输出参数是一个numpy数组或者一个numpy数组的列表,代表计算出来的直方图。
这个函数的实现方式与OpenCV中的calcHist函数类似,但是在一些细节上有所不同。例如,这个函数使用了numpy数组的方法来遍历像素并计算直方图。另外,这个函数不支持多通道图像的直方图计算。
python 重写calcHist底层代码
calcHist函数是OpenCV中用于计算图像直方图的函数,其实现是基于C++的。如果你想重写calcHist的底层代码,你需要了解OpenCV的底层实现和C++编程语言。
以下是一个简单的C++代码示例,用于计算一幅灰度图像的直方图:
```cpp
Mat image = imread("image.jpg");
Mat hist = Mat::zeros(256, 1, CV_32SC1);
for (int i = 0; i < image.rows; i++) {
for (int j = 0; j < image.cols; j++) {
int pixel = (int)image.at<uchar>(i, j);
hist.at<int>(pixel)++;
}
}
```
在上面的代码中,我们首先读取了一幅灰度图像,然后创建了一个256行1列的矩阵,用于存储直方图。接下来,我们使用两个嵌套的for循环遍历图像的每个像素,并将其像素值作为索引,递增hist矩阵中相应元素的值。
需要注意的是,上述代码仅仅是一个简单的示例,实际上OpenCV的calcHist函数实现要比这个复杂得多。如果你想要重写calcHist函数的底层代码,建议你深入学习OpenCV和C++编程语言。
相关推荐
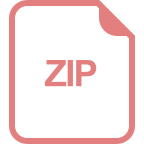
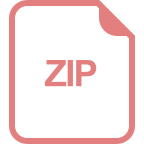
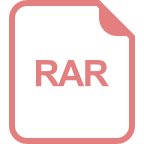




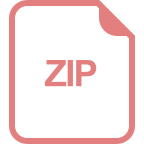
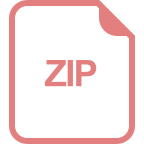