用C++实现以下全部问题
时间: 2024-12-17 21:26:59 浏览: 5
好的,以下是使用 C++ 实现斐波那契数列、Floyd 算法和 0/1 背包问题的代码示例:
### 1. 斐波那契数列
```cpp
#include <iostream>
#include <vector>
int fibonacci(int n) {
if (n <= 1) return n;
std::vector<int> dp(n + 1, 0);
dp[0] = 0;
dp[1] = 1;
for (int i = 2; i <= n; ++i) {
dp[i] = dp[i - 1] + dp[i - 2];
}
return dp[n];
}
int main() {
int n;
std::cout << "Enter the value of n: ";
std::cin >> n;
std::cout << "Fibonacci number at position " << n << " is " << fibonacci(n) << std::endl;
return 0;
}
```
### 2. Floyd 算法
```cpp
#include <iostream>
#include <vector>
#include <climits>
void floyd(std::vector<std::vector<int>>& graph, int V) {
std::vector<std::vector<int>> dist(graph);
// Initialize the distance matrix with the graph's edge weights
for (int i = 0; i < V; ++i) {
for (int j = 0; j < V; ++j) {
if (graph[i][j] == 0 && i != j) {
dist[i][j] = INT_MAX;
}
}
}
// Apply Floyd-Warshall algorithm
for (int k = 0; k < V; ++k) {
for (int i = 0; i < V; ++i) {
for (int j = 0; j < V; ++j) {
if (dist[i][k] != INT_MAX && dist[k][j] != INT_MAX && dist[i][k] + dist[k][j] < dist[i][j]) {
dist[i][j] = dist[i][k] + dist[k][j];
}
}
}
}
// Print the shortest path matrix
for (int i = 0; i < V; ++i) {
for (int j = 0; j < V; ++j) {
if (dist[i][j] == INT_MAX) {
std::cout << "INF ";
} else {
std::cout << dist[i][j] << " ";
}
}
std::cout << std::endl;
}
}
int main() {
int V;
std::cout << "Enter the number of vertices: ";
std::cin >> V;
std::vector<std::vector<int>> graph(V, std::vector<int>(V, 0));
std::cout << "Enter the adjacency matrix:\n";
for (int i = 0; i < V; ++i) {
for (int j = 0; j < V; ++j) {
std::cin >> graph[i][j];
}
}
floyd(graph, V);
return 0;
}
```
### 3. 0/1 背包问题
```cpp
#include <iostream>
#include <vector>
int knapsack(int W, const std::vector<int>& wt, const std::vector<int>& val, int n) {
std::vector<std::vector<int>> dp(n + 1, std::vector<int>(W + 1, 0));
for (int i = 1; i <= n; ++i) {
for (int w = 1; w <= W; ++w) {
if (wt[i - 1] + dp[i - 1][w - wt[i - 1]], dp[i - 1][w]);
} else {
dp[i][w] = dp[i - 1][w];
}
}
}
return dp[n][W];
}
int main() {
int n, W;
std::cout << "Enter the number of items: ";
std::cin >> n;
std::cout << "Enter the capacity of the knapsack: ";
std::cin >> W;
std::vector<int> val(n), wt(n);
std::cout << "Enter the values of the items:\n";
for (int i = 0; i < n; ++i) {
std::cin >> val[i];
}
std::cout << "Enter the weights of the items:\n";
for (int i = 0; i < n; ++i) {
std::cin >> wt[i];
}
int max_value = knapsack(W, wt, val, n);
std::cout << "The maximum value that can be obtained is: " << max_value << std::endl;
return 0;
}
```
这些代码分别实现了斐波那契数列、Floyd 算法和 0/1 背包问题,并且都使用了动态规划的方法。希望这些代码对你有帮助!如果有任何问题或需要进一步解释,请随时告诉我。
阅读全文
相关推荐



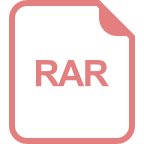
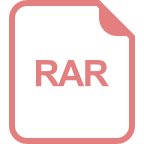
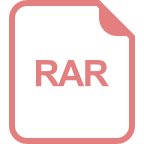
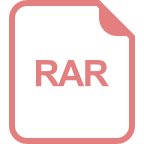
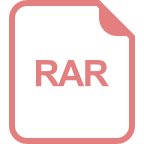
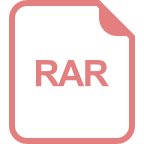
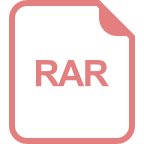
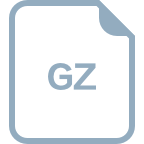


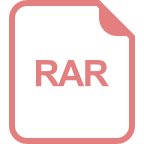
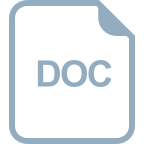
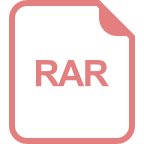

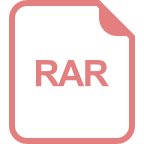
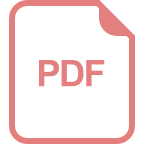