c++ wxwidgets 把int类型转为wxString类型
时间: 2024-09-15 08:04:28 浏览: 54
在C++的wxWidgets框架中,将int类型转换为wxString类型是一个简单的过程。你可以使用wxString类的构造函数或者ToString函数来实现这个转换。以下是具体的转换方法:
1. 使用构造函数直接转换:
```cpp
int myInt = 123;
wxString strInt = wxString::Format("%d", myInt);
```
这里使用了`wxString::Format`方法,它接受一个格式化的字符串,并返回一个新的wxString对象。
2. 使用ToString函数(如果你定义了自己的类并想重载ToString方法):
```cpp
class MyClass {
public:
int myInt;
virtual wxString ToString() const {
return wxString::Format("%d", myInt);
}
};
// 创建MyClass的实例并使用ToString方法转换
MyClass myClass;
myClass.myInt = 123;
wxString strInt = myClass.ToString();
```
这种方式允许你自定义对象转换为字符串的格式,非常灵活。
相关问题
c++ wxwidgets 获取本地时间,并转为wxstring
在C++中,如果你想要使用wxWidgets库获取本地时间和将其转换为`wxString`,你可以按照以下步骤操作:
首先,你需要包含相关的头文件:
```cpp
#include <wx/time.h>
#include <wx/timectrl.h>
#include <wx/string.h>
```
然后,在需要的时间点上,你可以创建一个`wxDateTime`对象来获取当前时间:
```cpp
wxDateTime currentDate = wxNow();
```
接着,可以将`wxDateTime`对象转换为`wxString`格式:
```cpp
std::stringstream timeStr;
timeStr << currentDate.FormatISO8601(); // 使用ISO 8601标准格式
wxString formattedTime(timeStr.str());
```
这里,`FormatISO8601()`函数用于生成一个常见的日期时间字符串,` wxString(timeStr.str())`则是将流转换为`wxString`。
如果你想显示这个时间在一个控件上,比如`wxTextCtrl`,你可以这样做:
```cpp
wxTextCtrl* timeCtrl = new wxTextCtrl(parent, -1, formattedTime);
```
c++ wxwidgets wxString类型转为std::vector<double>类型数据
在C++中使用wxWidgets库时,可能会遇到需要将`wxString`类型的数据转换为`std::vector<double>`类型的情况。`wxString`是wxWidgets库中用于字符串操作的一个类,而`std::vector<double>`是C++标准库中的动态数组容器,用于存储一系列的`double`类型的数值。
以下是一个将`wxString`转换为`std::vector<double>`的基本示例:
```cpp
#include <wx/string.h>
#include <vector>
#include <sstream>
#include <string>
std::vector<double> ConvertwxStringToVectorDouble(const wxString& wxstr) {
std::vector<double> result;
std::istringstream iss(wxstr.ToStdString());
double value;
while (iss >> value) {
result.push_back(value);
}
return result;
}
```
在这个示例中,首先包含了必要的头文件,然后定义了一个`ConvertwxStringToVectorDouble`函数,它接受一个`wxString`作为参数。使用`wxString`的`ToStdString`方法将其转换为`std::string`,然后使用`std::istringstream`来解析字符串中的数值,并将它们存储到`std::vector<double>`中。
注意:在实际使用中,这个函数假设`wxString`中包含了用空格或其他空白字符分隔的多个数值字符串。如果数值之间使用的是其他分隔符,则需要相应地调整解析逻辑。
阅读全文
相关推荐





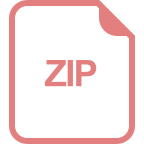









