用c语言写出对一个有N个学生的班级,每个学生有3门课程,分别为语文,英语,数学。该系统实现对班级成绩的录入、显示、修改、排序、保存等操作的管理。功能要求: (1)本系统采用一个结构体数组,每个数据的结构应当包括:学号、姓名、M门课程名称。 (2)本系统显示这样的菜单: 系统功能选项: a、 数据导入及显示 b、新记录输入 c、 成绩统计 1)显示每门课程成绩最高的学生的基本信息 2)显示每门课程的平均成绩 3)显示超过某门课程平均成绩的学生人数 g、 退出系统 注意:需保证学号唯一性 (该题目用数组完成)
时间: 2024-01-12 14:04:47 浏览: 27
以下是用C语言实现班级成绩管理系统的代码,其中包括录入、显示、修改、排序和保存等操作:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENT_NUM 100 // 最大学生数
#define COURSE_NUM 3 // 课程数
struct student {
int id; // 学号
char name[20]; // 姓名
float score[COURSE_NUM]; // 三门课程成绩
};
struct student stu[MAX_STUDENT_NUM]; // 学生数组
int student_num = 0; // 学生数
void input_scores();
void show_scores();
void modify_score();
void sort_by_score();
void save_scores();
void load_scores();
void print_menu();
int main() {
char choice;
load_scores(); // 读取之前保存的成绩
while (1) {
print_menu();
scanf("%c", &choice);
switch (choice) {
case 'a':
show_scores();
break;
case 'b':
input_scores();
break;
case 'c':
printf("1)显示每门课程成绩最高的学生的基本信息\n");
printf("2)显示每门课程的平均成绩\n");
printf("3)显示超过某门课程平均成绩的学生人数\n");
printf("请选择:");
scanf("%c", &choice);
switch (choice) {
case '1':
sort_by_score();
break;
case '2':
printf("语文平均成绩:%.2f\n", calc_average(0));
printf("英语平均成绩:%.2f\n", calc_average(1));
printf("数学平均成绩:%.2f\n", calc_average(2));
break;
case '3':
printf("请输入成绩:");
float score;
scanf("%f", &score);
printf("超过%.2f分的学生人数为:%d\n", score, count_above_score(score));
break;
default:
printf("无效选择!\n");
break;
}
break;
case 'g':
save_scores();
exit(0);
default:
printf("无效选择!\n");
break;
}
}
return 0;
}
// 录入学生成绩
void input_scores() {
printf("请输入学号(数字):");
int id;
scanf("%d", &id);
// 检查学号是否已经存在
for (int i = 0; i < student_num; i++) {
if (stu[i].id == id) {
printf("该学号已存在!\n");
return;
}
}
printf("请输入姓名:");
char name[20];
scanf("%s", name);
// 录入三门课程成绩
float score[COURSE_NUM];
for (int i = 0; i < COURSE_NUM; i++) {
printf("请输入%s成绩:", (i == 0) ? "语文" : (i == 1) ? "英语" : "数学");
scanf("%f", &score[i]);
}
// 添加新学生
stu[student_num].id = id;
strcpy(stu[student_num].name, name);
memcpy(stu[student_num].score, score, sizeof(score));
student_num++;
printf("学生成绩录入成功!\n");
}
// 显示所有学生成绩
void show_scores() {
if (student_num == 0) {
printf("没有学生成绩!\n");
return;
}
printf("学号\t姓名\t语文\t英语\t数学\n");
for (int i = 0; i < student_num; i++) {
printf("%d\t%s\t%.2f\t%.2f\t%.2f\n", stu[i].id, stu[i].name, stu[i].score[0], stu[i].score[1], stu[i].score[2]);
}
}
// 修改学生成绩
void modify_score() {
printf("请输入学号:");
int id;
scanf("%d", &id);
for (int i = 0; i < student_num; i++) {
if (stu[i].id == id) {
printf("请输入要修改的科目(0:语文,1:英语,2:数学):");
int course;
scanf("%d", &course);
printf("请输入新成绩:");
float score;
scanf("%f", &score);
stu[i].score[course] = score;
printf("学生成绩修改成功!\n");
return;
}
}
printf("没有找到该学生!\n");
}
// 按成绩排序并显示
void sort_by_score() {
printf("请选择要排序的科目(0:语文,1:英语,2:数学):");
int course;
scanf("%d", &course);
// 冒泡排序
for (int i = 0; i < student_num - 1; i++) {
for (int j = 0; j < student_num - i - 1; j++) {
if (stu[j].score[course] < stu[j + 1].score[course]) {
struct student temp = stu[j];
stu[j] = stu[j + 1];
stu[j + 1] = temp;
}
}
}
printf("排名\t学号\t姓名\t成绩\n");
for (int i = 0; i < student_num; i++) {
printf("%d\t%d\t%s\t%.2f\n", i + 1, stu[i].id, stu[i].name, stu[i].score[course]);
}
}
// 计算某门课程的平均成绩
float calc_average(int course) {
float sum = 0;
for (int i = 0; i < student_num; i++) {
sum += stu[i].score[course];
}
return sum / student_num;
}
// 计算某门课程成绩超过指定分数的学生人数
int count_above_score(float score) {
int count = 0;
for (int i = 0; i < student_num; i++) {
if (stu[i].score[0] > score || stu[i].score[1] > score || stu[i].score[2] > score) {
count++;
}
}
return count;
}
// 保存成绩到文件
void save_scores() {
FILE *fp = fopen("scores.dat", "wb");
if (fp == NULL) {
printf("文件保存失败!\n");
return;
}
fwrite(&student_num, sizeof(int), 1, fp);
fwrite(stu, sizeof(struct student), student_num, fp);
fclose(fp);
printf("成绩保存成功!\n");
}
// 从文件中读取成绩
void load_scores() {
FILE *fp = fopen("scores.dat", "rb");
if (fp == NULL) {
printf("没有找到之前保存的成绩!\n");
return;
}
fread(&student_num, sizeof(int), 1, fp);
fread(stu, sizeof(struct student), student_num, fp);
fclose(fp);
printf("成绩读取成功!\n");
}
// 输出菜单
void print_menu() {
printf("系统功能选项:\n");
printf("a、数据导入及显示\n");
printf("b、新记录输入\n");
printf("c、成绩统计\n");
printf("g、退出系统\n");
printf("请选择:");
fflush(stdin);
}
```
相关推荐
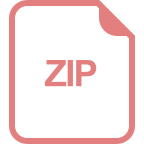



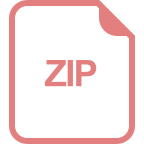
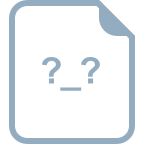
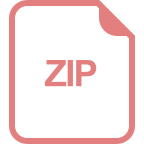
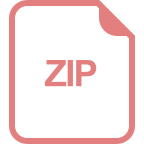
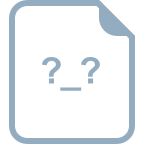
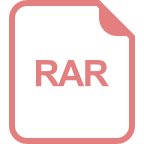
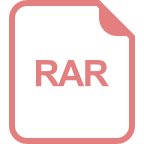
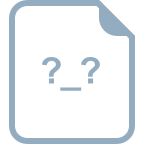