用Java代码编写一个程序实现一下功能 1)从已有的图象文件列表中选择某一个,即可显示该图象,并可做旋转等变换。 2)在画布区域上可以进行各种规则图形的绘制(如:线段、矩形、多边形、圆等),还可通过鼠标的拖动自由绘图。 3)可以连续播放所选文件夹下的所有图象。
时间: 2024-03-11 16:45:34 浏览: 58
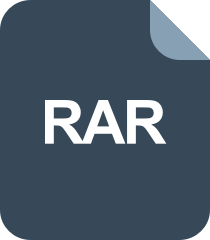
java实现的一个画板小程序画直线,矩形,三角形以及拖动图形功能

好的,以下是Java代码实现上述功能的示例:
1. 显示图像并进行变换:
```java
import java.awt.*;
import java.awt.event.*;
import java.awt.geom.AffineTransform;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.*;
public class ImageDisplay extends JFrame implements ActionListener {
private JLabel imageLabel;
private BufferedImage image;
private JComboBox<String> imageList;
private JCheckBox rotateBox;
private JButton rotateButton;
private double angle = 0.0;
public ImageDisplay() {
super("Image Display");
JPanel controlPanel = new JPanel();
controlPanel.setLayout(new FlowLayout());
JLabel label = new JLabel("Select Image:");
controlPanel.add(label);
// 添加下拉列表框用于选择图像文件
imageList = new JComboBox<String>(new String[]{"image1.jpg", "image2.jpg", "image3.jpg"});
imageList.addActionListener(this);
controlPanel.add(imageList);
// 添加复选框用于控制是否旋转
rotateBox = new JCheckBox("Rotate");
rotateBox.addActionListener(this);
controlPanel.add(rotateBox);
// 添加按钮用于执行旋转操作
rotateButton = new JButton("Rotate");
rotateButton.addActionListener(this);
controlPanel.add(rotateButton);
// 将控制面板添加到窗口的南侧
add(controlPanel, BorderLayout.SOUTH);
// 读取默认的图像文件并将其显示
try {
image = ImageIO.read(new File(imageList.getSelectedItem().toString()));
imageLabel = new JLabel(new ImageIcon(image));
add(imageLabel, BorderLayout.CENTER);
} catch (IOException e) {
e.printStackTrace();
}
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(800, 600);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
Object source = e.getSource();
if (source == imageList) {
// 当下拉列表框的选项改变时,读取新的图像文件并显示
try {
image = ImageIO.read(new File(imageList.getSelectedItem().toString()));
imageLabel.setIcon(new ImageIcon(image));
angle = 0.0;
} catch (IOException ex) {
ex.printStackTrace();
}
} else if (source == rotateBox || source == rotateButton) {
// 当复选框或按钮被选中时,旋转图像
if (rotateBox.isSelected()) {
angle += Math.PI / 4.0;
} else {
angle -= Math.PI / 4.0;
}
// 使用 AffineTransform 对图像进行变换
AffineTransform transform = new AffineTransform();
transform.translate(image.getWidth() / 2.0, image.getHeight() / 2.0);
transform.rotate(angle);
transform.translate(-image.getWidth() / 2.0, -image.getHeight() / 2.0);
BufferedImage rotatedImage = new BufferedImage(image.getWidth(), image.getHeight(), image.getType());
Graphics2D g = rotatedImage.createGraphics();
g.drawImage(image, transform, null);
g.dispose();
imageLabel.setIcon(new ImageIcon(rotatedImage));
}
}
public static void main(String[] args) {
new ImageDisplay();
}
}
```
2. 绘制图形:
```java
import java.awt.*;
import java.awt.event.*;
import java.awt.geom.*;
import javax.swing.*;
public class DrawingApplication extends JFrame implements MouseListener, MouseMotionListener, ActionListener {
private JPanel canvas;
private JButton lineButton, rectButton, ellipseButton, polygonButton, freehandButton;
private Shape currentShape;
private Point startPoint, endPoint;
private Polygon currentPolygon;
public DrawingApplication() {
super("Drawing Application");
// 创建绘图区域
canvas = new JPanel() {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
if (currentShape != null) {
((Graphics2D) g).draw(currentShape);
}
if (currentPolygon != null) {
((Graphics2D) g).drawPolygon(currentPolygon);
}
}
};
canvas.setBackground(Color.WHITE);
canvas.addMouseListener(this);
canvas.addMouseMotionListener(this);
add(canvas, BorderLayout.CENTER);
// 创建绘图工具栏
JPanel toolBar = new JPanel();
toolBar.setLayout(new FlowLayout());
lineButton = new JButton("Line");
lineButton.addActionListener(this);
toolBar.add(lineButton);
rectButton = new JButton("Rectangle");
rectButton.addActionListener(this);
toolBar.add(rectButton);
ellipseButton = new JButton("Ellipse");
ellipseButton.addActionListener(this);
toolBar.add(ellipseButton);
polygonButton = new JButton("Polygon");
polygonButton.addActionListener(this);
toolBar.add(polygonButton);
freehandButton = new JButton("Freehand");
freehandButton.addActionListener(this);
toolBar.add(freehandButton);
add(toolBar, BorderLayout.NORTH);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(800, 600);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
Object source = e.getSource();
if (source == lineButton) {
currentShape = null;
currentPolygon = null;
canvas.repaint();
JOptionPane.showMessageDialog(this, "Click and drag to draw a line");
} else if (source == rectButton) {
currentShape = null;
currentPolygon = null;
canvas.repaint();
JOptionPane.showMessageDialog(this, "Click and drag to draw a rectangle");
} else if (source == ellipseButton) {
currentShape = null;
currentPolygon = null;
canvas.repaint();
JOptionPane.showMessageDialog(this, "Click and drag to draw an ellipse");
} else if (source == polygonButton) {
currentShape = null;
currentPolygon = new Polygon();
canvas.repaint();
JOptionPane.showMessageDialog(this, "Click to add points to the polygon, and double-click to finish");
} else if (source == freehandButton) {
currentShape = null;
currentPolygon = null;
canvas.repaint();
JOptionPane.showMessageDialog(this, "Hold down the mouse button to draw a freehand curve");
}
}
@Override
public void mouseClicked(MouseEvent e) {
if (currentPolygon != null) {
if (e.getClickCount() == 2) {
currentShape = new Polygon(currentPolygon.xpoints, currentPolygon.ypoints, currentPolygon.npoints);
currentPolygon = null;
canvas.repaint();
} else {
currentPolygon.addPoint(e.getX(), e.getY());
canvas.repaint();
}
}
}
@Override
public void mousePressed(MouseEvent e) {
startPoint = e.getPoint();
}
@Override
public void mouseReleased(MouseEvent e) {
endPoint = e.getPoint();
if (currentShape == null) {
if (lineButton.isSelected()) {
currentShape = new Line2D.Double(startPoint, endPoint);
} else if (rectButton.isSelected()) {
currentShape = new Rectangle2D.Double(Math.min(startPoint.x, endPoint.x), Math.min(startPoint.y, endPoint.y),
Math.abs(endPoint.x - startPoint.x), Math.abs(endPoint.y - startPoint.y));
} else if (ellipseButton.isSelected()) {
currentShape = new Ellipse2D.Double(Math.min(startPoint.x, endPoint.x), Math.min(startPoint.y, endPoint.y),
Math.abs(endPoint.x - startPoint.x), Math.abs(endPoint.y - startPoint.y));
}
} else {
currentShape = null;
}
canvas.repaint();
}
@Override
public void mouseEntered(MouseEvent e) {
}
@Override
public void mouseExited(MouseEvent e) {
}
@Override
public void mouseDragged(MouseEvent e) {
if (freehandButton.isSelected()) {
Line2D line = new Line2D.Double(startPoint, e.getPoint());
currentShape = line;
startPoint = e.getPoint();
canvas.repaint();
}
}
@Override
public void mouseMoved(MouseEvent e) {
}
public static void main(String[] args) {
new DrawingApplication();
}
}
```
3. 连续播放图像:
```java
import java.awt.*;
import java.awt.event.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.*;
public class ImagePlayer extends JFrame implements ActionListener {
private JLabel imageLabel;
private BufferedImage currentImage;
private Timer timer;
private int currentImageIndex;
private File[] imageFiles;
public ImagePlayer() {
super("Image Player");
// 选择图像文件夹
JFileChooser fileChooser = new JFileChooser();
fileChooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
int result = fileChooser.showOpenDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
// 读取图像文件夹下的所有图像文件
File imageFolder = fileChooser.getSelectedFile();
imageFiles = imageFolder.listFiles((dir, name) -> name.endsWith(".jpg") || name.endsWith(".png"));
} else {
JOptionPane.showMessageDialog(this, "Please select an image folder");
System.exit(0);
}
// 创建显示图像的标签
imageLabel = new JLabel();
add(imageLabel, BorderLayout.CENTER);
// 创建计时器并启动
timer = new Timer(1000, this);
timer.start();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(800, 600);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
// 切换到下一张图像
currentImageIndex = (currentImageIndex + 1) % imageFiles.length;
try {
currentImage = ImageIO.read(imageFiles[currentImageIndex]);
imageLabel.setIcon(new ImageIcon(currentImage));
} catch (IOException ex) {
ex.printStackTrace();
}
}
public static void main(String[] args) {
new ImagePlayer();
}
}
```
阅读全文
相关推荐
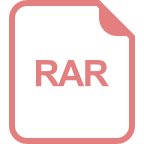
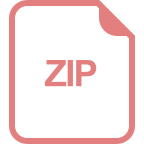

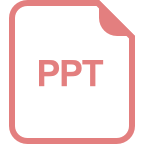
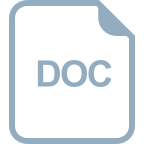
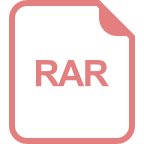
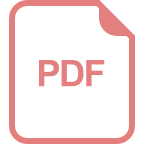
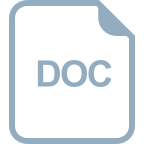
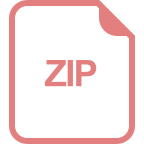
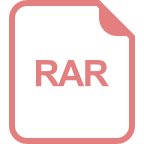
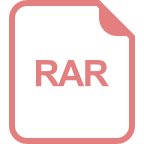
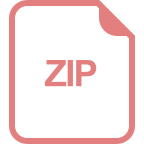
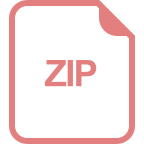
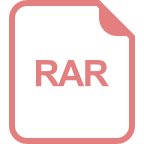