js格式化时间为‘YYYY-MM-DD’
时间: 2024-10-15 20:21:04 浏览: 20
在JavaScript中,你可以使用`Date`对象的内置方法来将时间格式化为'YYYY-MM-DD'的字符串形式。下面是一个简单的例子:
```javascript
function formatDate(date) {
const year = date.getFullYear();
const month = ('0' + (date.getMonth() + 1)).slice(-2); //注意月份从0开始计数,加1
const day = ('0' + date.getDate()).slice(-2);
return `${year}-${month}-${day}`;
}
// 使用当前日期示例
const today = new Date();
const formattedDate = formatDate(today);
console.log(formattedDate); // 输出类似 "2023-04-25"
```
在这个函数中,我们首先获取年份、月份(注意加上1因为getMonth返回的是0到11),然后使用`slice(-2)`确保始终是两位数,最后将它们拼接成所需的格式。
如果你需要用户输入的时间,记得检查`date.getMonth()`是否在正确的范围内(0-11),并处理可能出现的问题。
相关问题
JS格式化时间为yyyy-mm-dd格式
可以使用 JavaScript 中的 Date 对象来格式化时间。以下是将当前时间格式化为 yyyy-mm-dd 格式的示例代码:
```javascript
const now = new Date();
const year = now.getFullYear();
const month = ('0' + (now.getMonth() + 1)).slice(-2);
const day = ('0' + now.getDate()).slice(-2);
const formattedDate = year + '-' + month + '-' + day;
console.log(formattedDate); // 输出当前日期的 yyyy-mm-dd 格式
```
如果要格式化指定的时间,可以将时间字符串或时间戳作为参数传递给 `new Date()`。例如,要将时间戳 `1623896100000` 格式化为 yyyy-mm-dd 格式:
```javascript
const timestamp = 1623896100000;
const date = new Date(timestamp);
const year = date.getFullYear();
const month = ('0' + (date.getMonth() + 1)).slice(-2);
const day = ('0' + date.getDate()).slice(-2);
const formattedDate = year + '-' + month + '-' + day;
console.log(formattedDate); // 输出 2021-06-17
```
js 时间格式化 yyyy-MM-dd
您可以使用以下代码进行 JS 时间格式化:
```
function formatDate(date) {
const year = date.getFullYear()
const month = (date.getMonth() + 1).toString().padStart(2, '0')
const day = date.getDate().toString().padStart(2, '0')
return year + '-' + month + '-' + day
}
// 使用示例
const date = new Date('2022/01/18')
console.log(formatDate(date)) // 输出 2022-01-18
```
希望对您有帮助!如果您有其它问题,请随时问我。
阅读全文
相关推荐
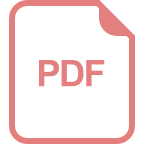
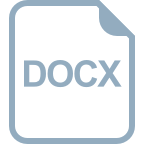
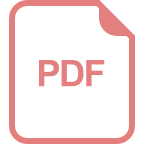













