贪心法付款算法代码c++
时间: 2024-11-28 08:15:31 浏览: 11
贪心法是一种常用的算法设计策略,它每一步都选择当前看起来最优的解决方案,希望最终能得出全局最优解。在付款算法中,例如计算给定商品价格列表最优惠的购买组合,可以使用贪心法通过优先选择单价最低的商品,直到总金额不超过预算。
下面是一个简单的C++贪心法付款算法示例,假设我们有一个商品列表,每个商品都有一个价格和数量:
```cpp
#include <iostream>
#include <vector>
#include <algorithm> // 用于sort
// 定义商品结构体
struct Product {
int price;
int quantity;
};
bool compareProducts(const Product &a, const Product &b) { // 按照价格升序排序
return a.price < b.price;
}
int greedyPaymentAlgorithm(std::vector<Product>& products, int budget) {
std::sort(products.begin(), products.end(), compareProducts); // 排序商品
int totalCost = 0;
for (const auto &product : products) {
if (totalCost + product.price <= budget) { // 如果剩余预算足够
totalCost += product.price;
product.quantity--;
} else { // 否则,只能买下一个最小单位
totalCost += product.price * product.quantity;
break;
}
}
return totalCost;
}
int main() {
std::vector<Product> products = {{50, 2}, {30, 3}, {70, 1}}; // 商品列表
int budget = 100; // 预算
int optimalCost = greedyPaymentAlgorithm(products, budget);
std::cout << "Optimal cost: " << optimalCost << std::endl;
return 0;
}
```
在这个例子中,程序首先对商品按价格从低到高排序,然后遍历商品,每次尽可能多地购买价格最低的商品,直到预算不足为止。注意这只是一个基本的贪心算法实现,并非所有情况都能保证得到全局最优解,因为可能存在某些特定条件下,非贪婪策略才能找到更优方案。
阅读全文
相关推荐
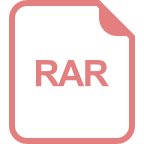
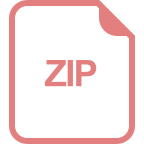
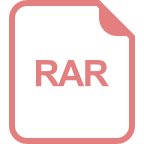
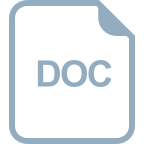
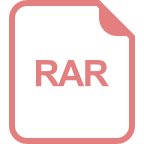
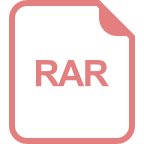
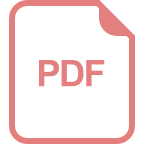
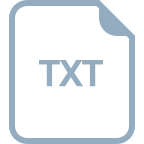
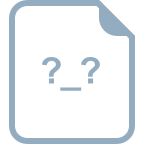
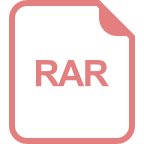
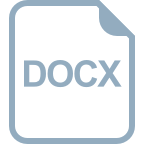
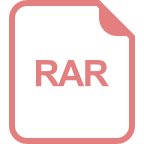