TypeError: super() argument 1 must be a type, not Maxpool
时间: 2024-09-24 18:29:49 浏览: 53
这个错误通常发生在Python的继承体系中,当你试图使用`super()`函数创建一个新的实例,但传递给它的第一个参数不是一个类型(即不是父类或其基类),而是实例本身(在这个例子中是`Maxpool`)。`super()`函数用于调用父类的方法,它期望的第一个参数是一个类,表示你要向上查找的对象的超类。
例如,在Python的类定义中,如果你有:
```python
class Parent:
def __init__(self):
super().__init__()
class Maxpool(Parent): # 错误的地方
pass
maxpool_instance = Maxpool()
```
这里`maxpool_instance`是一个`Maxpool`实例而不是类,所以在`__init__`方法中调用`super().__init__()`会抛出TypeError。
修复这个问题,你应该确保`Maxpool`类的构造函数正确地调用了父类的构造函数,应该像这样:
```python
class Maxpool(Parent):
def __init__(self):
super(Maxpool, self).__init__() # 更正后的调用
```
或者去掉`super()`,如果`Parent`类没有明确需要初始化的行为:
```python
class Maxpool(Parent):
pass
```
相关问题
Python super(Todo,self).__init__() TypeError: super() argument 1 must be type, not classobj
这个错误通常是由于 Python 2 和 Python 3 中 `super()` 函数的使用方式不同导致的。
在 Python 2 中,`super()` 函数需要两个参数:第一个参数是当前类,第二个参数是当前类的实例。而在 Python 3 中,`super()` 函数只需要一个参数,即当前类,因为 Python 3 中可以通过类名自动获取当前实例。
如果你的代码是在 Python 2 中编写的,可以使用以下方式来调用 `super()` 函数:
```python
super(Todo, self).__init__()
```
如果你的代码是在 Python 3 中编写的,可以直接使用以下方式来调用 `super()` 函数:
```python
super().__init__()
```
这样就可以避免出现该错误了。
TypeError: layer_norm(): argument 'input' (position 1) must be Tensor, not DataFrame
这个错误是因为您在LayerNorm层中传递了DataFrame类型的数据,但是LayerNorm层只支持Tensor类型的数据作为输入。您需要先将DataFrame类型的数据转换为Tensor类型,然后再传递给LayerNorm层。
您可以将DataFrame类型的数据转换为Numpy数组,然后再将Numpy数组转换为Tensor类型,具体操作如下:
```python
train_x = torch.tensor(df1.values, dtype=torch.float32)
train_y = torch.tensor(df2.values, dtype=torch.float32)
```
在上面的代码中,我们使用DataFrame的values属性将DataFrame类型的数据转换为Numpy数组,然后使用torch.tensor将Numpy数组转换为Tensor类型,以便在神经网络中使用。
在您的代码中,您需要将train_x和train_y都转换为Tensor类型,然后再传递给LayerNorm层。具体操作如下:
```python
train_x = torch.tensor(df1.values, dtype=torch.float32)
train_y = torch.tensor(df2.values, dtype=torch.float32)
train_y = train_y.unsqueeze(1)
# 定义模型
class Wine_net(nn.Module):
def __init__(self):
super(Wine_net, self).__init__()
self.ln1 = nn.LayerNorm(11)
self.fc1 = nn.Linear(11, 22)
self.fc2 = nn.Linear(22, 44)
self.fc3 = nn.Linear(44, 1)
def forward(self, x):
x = self.ln1(x)
x = self.fc1(x)
x = nn.functional.relu(x)
x = self.fc2(x)
x = nn.functional.relu(x)
x = self.fc3(x)
x = nn.functional.softmax(x, dim=1)
return x
# 定义损失函数和优化器
model = Wine_net()
loss_fn = nn.MSELoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.1)
# 训练模型
for epoch in range(10):
# 前向传播
y_pred = model(train_x)
# 计算损失
loss = loss_fn(y_pred, train_y)
# 反向传播
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 打印损失
print('Epoch:', epoch, 'Train Loss:', loss.item())
```
在上面的代码中,我们首先将train_x和train_y都转换为Tensor类型,并使用unsqueeze方法将train_y的维度从[样本数,]转换为[样本数, 1],以便在计算损失时能够与y_pred正确地对齐。然后我们定义了模型、损失函数和优化器,并在每次迭代中前向传播、计算损失、反向传播、更新模型参数并打印损失。
阅读全文
相关推荐
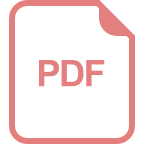
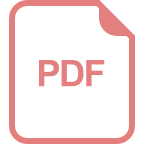

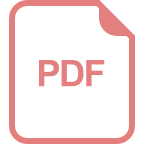
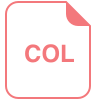
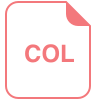
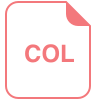
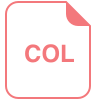
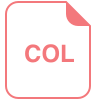
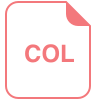


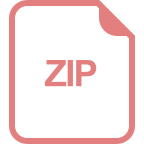
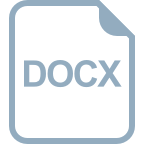
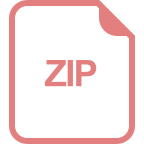
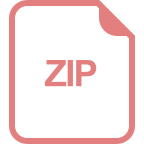